Render Reference - Render Targets
Render Library References
These pages seek to provide helpful insight into the groups of functions provided by the Render Library.
The major function groups are:
- Beams
- Minification and Magnification Texture Filters
- Render Targets
What are Render Targets?
A Render Target is a special kind of texture that is created during gameplay via the game's code, rather than from a pre-existing image that is loaded from the game's files. Like other textures, Render Targets can be drawn as 2D images, like a HUD element, or as the base texture for Materials to be used on 3D objects.
Render Targets have a fixed resolution, measured in pixels, that is defined when they are first created.
Unless your specific use-case has a reason not to, it's recommended to use the game's render resolution (Obtained via ScrW and ScrH.)
Render Targets have 3 layers:
- The Color Channel (or simply "Color")
- This is what will be seen when the Render Target is drawn onto the screen.
- Each pixel in the Render Target's Color Channel has a red, green, blue, and alpha value.
- The Depth Buffer (or simply "Depth")
- This layer keeps track of how far away from the camera each pixel in the Render Target is.
- Each pixel in the Depth Buffer has a whole, integer number between 0-255 where 0 is close to the camera and 255 is far from the camera.
- The Stencil Buffer (or simply "Stencils")
- The Stencil layer controls whether or not each individual pixel in the Render Target is able to be modified by drawing and rendering operations.
- Each pixel in the Stencil buffer has a whole, integer number between 0-255.
- By default, the Stencil layer is configured so that all pixels can be drawn to.
What are Practical Uses for Render Targets?
While this is not a complete or exhaustive list, here are some examples of situations where a Render Target would be useful:
- A rear-view mirror for a vehicle.
- A security camera feed shown on a TV in a building's security room.
- A painting canvas that players can draw on.
The Game's Main View is a Render Target
It's important to understand that Render Targets are a core piece of the game's rendering system.
Any drawing or rendering operation that you can do to the game's main view can be done to a Render Target because they are both Render Targets.
The Source Engine, and by extension Garry's Mod, uses a "double buffered" rendering system. This means the game creates two Render Targets (Called "Frame Buffers" when used for this purpose.) One of these Render Targets is the "Front" and one is the "Back". The Front Render Target is what gets drawn onto the screen for the player to see. Meanwhile, the next frame of the game gets drawn onto the Back Render Target. Once the frame has been fully drawn onto the Back Render Target, the rendering system swaps (Called "Spinning") the Front and Back Render Targets and the process starts again.
Example Code
Example
The following example code should provide a good demonstration of how to set up a basic Render Target, how to draw 2D and 3D elements onto it, and then how to draw the Render Target onto the screen.
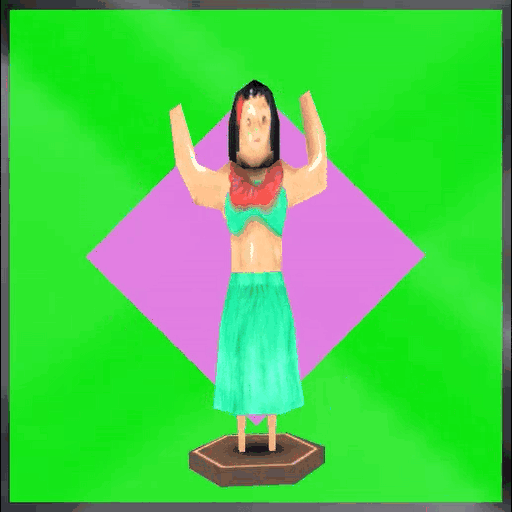