AnimEvents, AnimGraph Tags, Attachments
What are AnimEvents?
AnimEvents are used in ModelDoc as a way to make something happen during a specific frame of a Simple Animation, most commonly used to:
What are AnimGraph Tags?
Animgraph Tags are used inside an AnimGraph to communicate with itself. You can specify the start and end of when a Tag is active inside individual Animation Clips, most commonly used to:
Attachments
An Attachment is a point, similar to bones, but created in ModelDoc, and is required for many functions that need a position, like most AnimEvents.
Creating Attachments in ModelDoc:
1. Press ✙add, add an Attachment
and name it.
2. Select which Bone will be its parent, this is the bone it will follow.
3. Position the attachment (you can use the various gizmos in the top left to aid you).
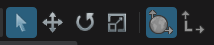
This should be the final result (mouth is the attachment):
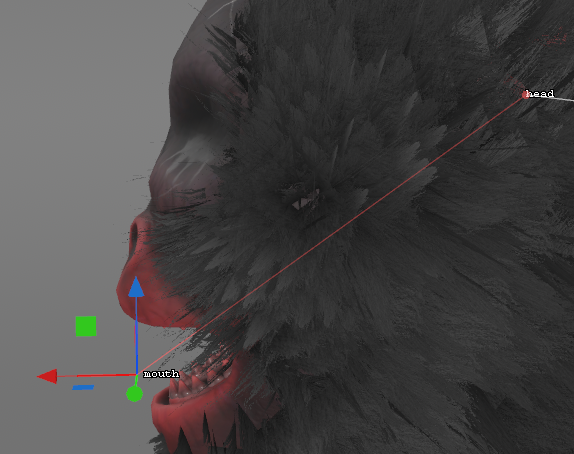
RED
arrow indicates forward, while the BLUE arrow is up, make sure they are correct by rotating the attachment in the node itself.AnimEvents
To add an AnimEvent Right Click a Simple Animation node, and select Add AnimEvent...
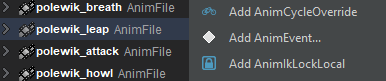
Once you selected an AnimEvent, you can choose the frame it will activate either in the Timeline, by dragging the AnimEvent, or in the Event's node by typing the frame.
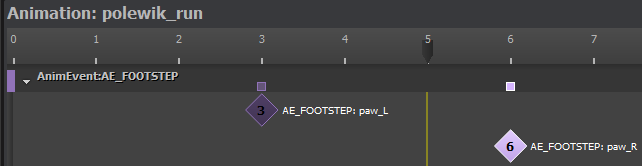
Sound Events
To play a sound, select:
- AE_CL_PLAYSOUND: Play a sound Clientside from the model's origin.
- AE_CL_PLAYSOUND_ATTACHMENT: Play a sound ClientSide from an Attachment.
- AE_SV_PLAYSOUND: Play a sound Serverside from the model's origin.
Then select a sound, after compiling, you can preview the AnimEvent:
Particle Events
- AE_CL_CREATE_PARTICLE_EFFECT: Create a particle from an Attachment.
- AE_CL_ADD_PARTICLE_EFFECT_CP: Add a Control Point for a Particle from an Attachment.
Then select a particle, and leave the default attachment type as is, as it should be fine for most cases. After compiling, you can preview the AnimEvent:
BodyGroup Events
To switch bodygroups, select:
- AE_CL_BODYGROUP_SET_VALUE: Set the Value of a bodygroup, clientside.
- AE_SV_BODYGROUP_SET_VALUE: Set the Value of a bodygroup, Serverside.
- AE_CL_ENABLE_BODYGROUP: Enable a bodygroup
- AE_CL_DISABLE_BODYGROUP: Disable a bodygroup
Then Select a bodygroup, and the desired value (equivalent to their "Choice" number, starting from 0). After compiling, you can preview the AnimEvent:
FootStep Events
To add dynamic footsteps, select:
- AE_FOOTSTEP: Play a dynamic Footstep sound from an Attachment
You may set the Volume of the footstep, and whether it's LEFT or RIGHT (Even if your character has more than 2 feet).
This example code is used for footsteps by checking the surface type below.
Run code with Generic Events
You may also add Generic Events that do nothing, but signal your game when the animation has an event.
AE_GENERIC_EVENT can include:
- Integer data
- Float data
- Vector data
- String data
- Type Name
AnimGraph Tags
To add a Tag in your Animgraph:
- In the Tags widget, press ✙ and select
Internal Tag
, name it accordingly.
Then, to set the Tag inside your Animation Clips:
- Double Click an Animation Clip to enter it.
- Press ✙ and select your Tag
- Slide the Tag's start and end for the desired duration
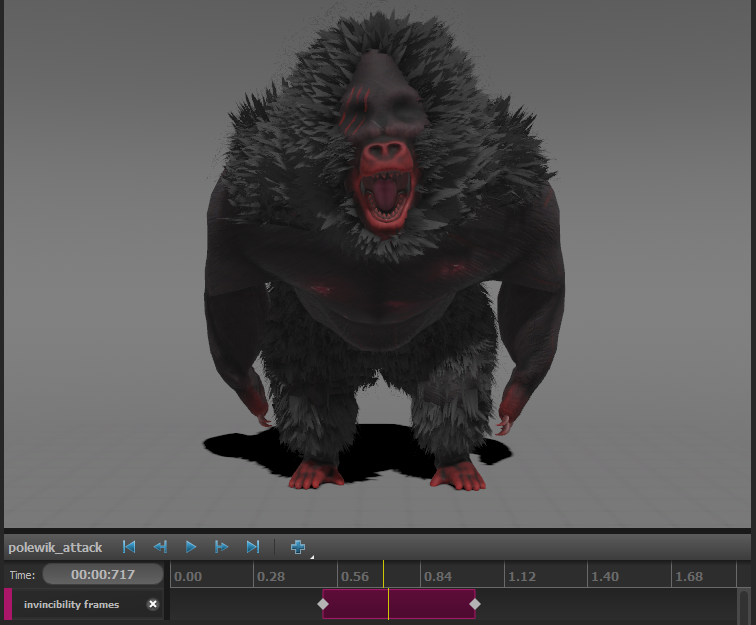
In this example, the "Invincibility Frames" tag lasts from 0.5s to 1.0s
Tag Condition in a State Machine
Inside a state machine, you can select Internal Tags as Tag Condition
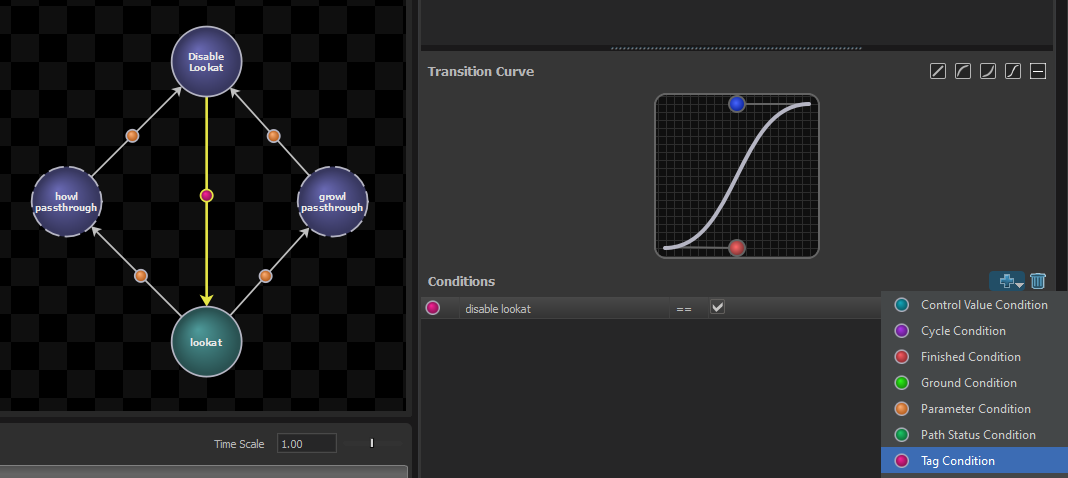
In this example, the "Disable LookAt" tag is used to disable a LookAt chain at the start of either "Growl" or "Howl" animations, until they finish their respective action.
Run Code with Tags
You can also use Tags to run code:
This example code will make a character invincible during the Invincibility Frames
tag, useful for features such as a Dark Souls-Styled Roll mechanic.