Input System
Input
Input is handled using the Input
class.
Input Actions
You can configure Input Actions for your project in the Project Settings.
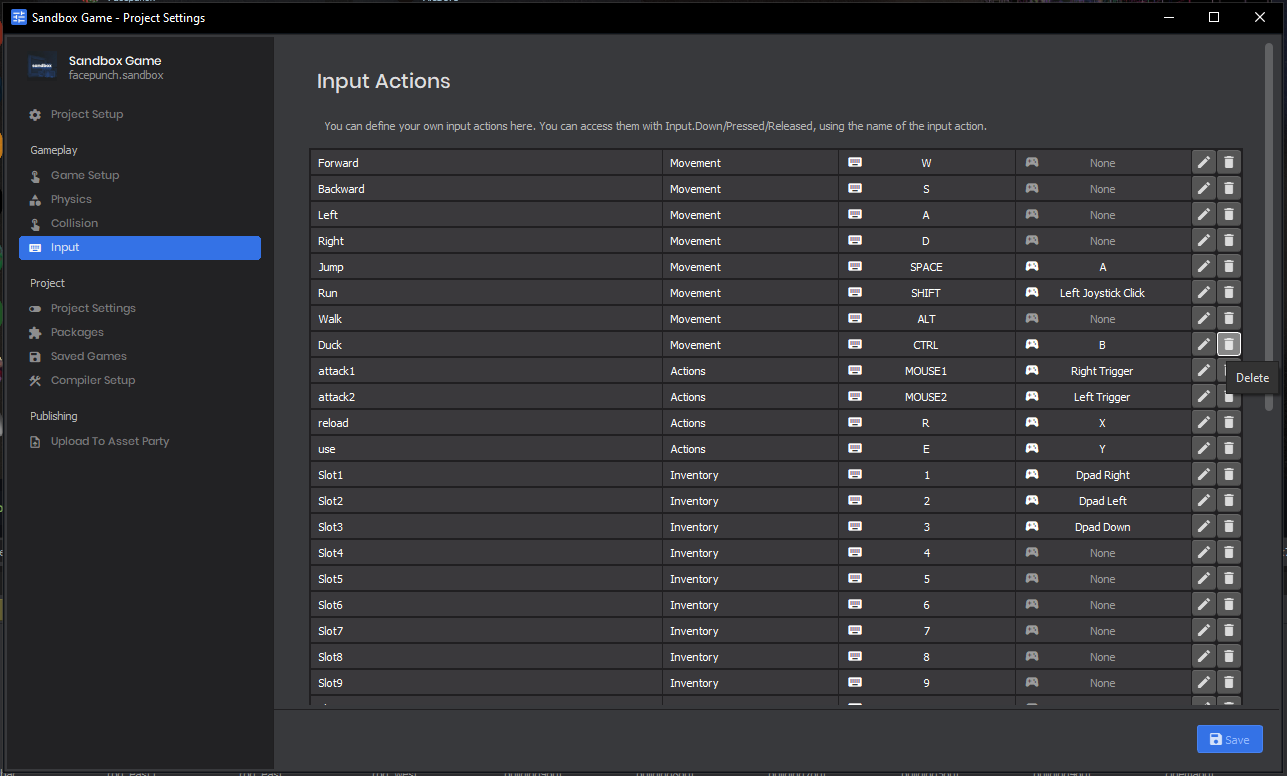
Using Actions
The Input class has a number of methods to help you use your input actions.
Method | Description |
---|---|
Input.Pressed( "jump" ) | input was just pressed this frame |
Input.Released( "jump" ) | input was just released this frame |
Input.Down( "jump" ) | input was held down |
It is common to query for input within OnUpdate
or OnFixedUpdate
.
AnalogMove
Input.AnalogMove
is generated automatically from controller input and any actions you have named "forward", "backward", "left" and "right".
This is a Vector3
representing the move input.
You can safely use this for something like player movement input but you should sanity check it first. For example, you might want to normalize it.
AnalogLook
Input.AnalogLook
is generated automatically from controller and mouse input.
This represents the view angles. This could be used in something like a first person shooter to represent the eye angles or rotation of a player's object.
MouseWheel
Input.Mousewheel
is a Vector2 to represent the scroll wheel. This is a Vector2 because many mouses have horizontal and vertical scrolling.
Input Glyphs
Input glyphs are an easy way for users to understand which buttons need to be pressed for actions to occur.
The following code is used to generate one:
Texture JumpButton = Input.GetGlyph( "jump" );
You can also choose if the glyph is outlined:
Texture JumpButton = Input.GetGlyph( "jump", true );
Input glyphs are automatically changed based on the player's input method, so it's worth generating them every frame.
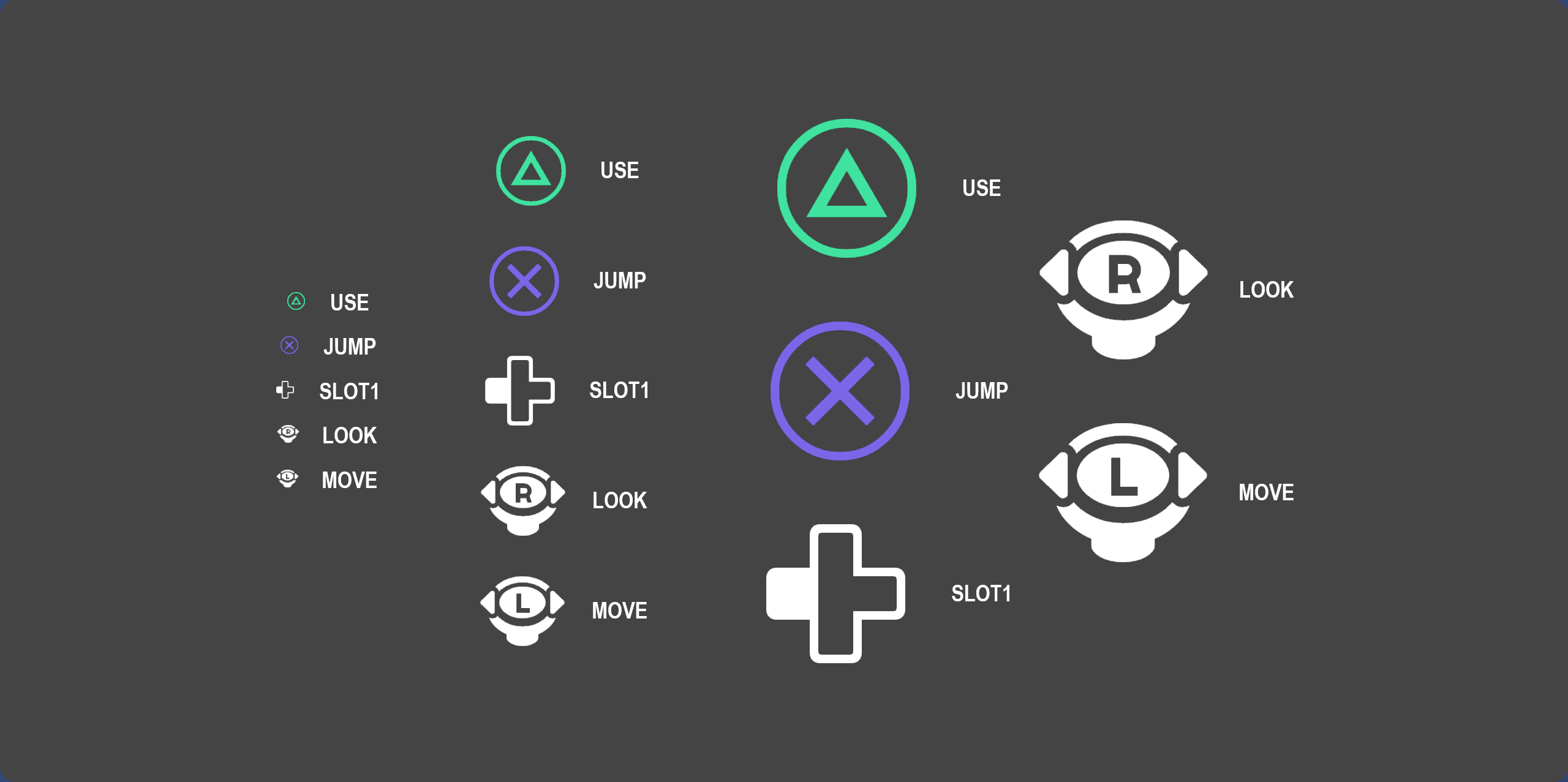
Razor Example
This will get a new texture each UI update, based on the user's "attack2" key.