GameObjects
GameObjects
GameObjects are the fundamental building blocks of a scene. A GameObject can have Components which can provide a wide variety of functionality.
Transform
You can manipulate a GameObject through code, including its Transform. A GameObject's Transform is held relative to its parent.
Tags
GameObjects have tags, which can be used a variety of ways. Some common usages include only returning GameObjects from a trace that include or omit certain tags, or determining what objects you want a specific camera to render.
You can add/remove tags in the Editor at the top of the Inspector for that GameObject. To add a new tag, click the tag+ icon and then pick an existing one or type the name of a new tag and hit Enter.
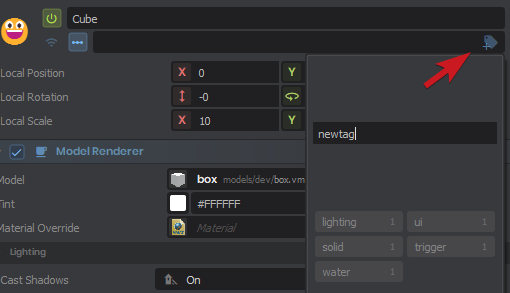
Directory
If you want to find a GameObject in your scene by name or Guid, you can use Scene.Directory
. You can also query Scene.GetAllObjects( bool enabled )
. These are expensive, so you shouldn't do it all the time (such as in OnUpdate).
As a Property
Sometimes, a Component may need a reference to a particular GameObject in your scene. You can set it as a property, and then reference it without having to iterate through all GameObjects in the scene to find it.