Shader Reference
Variables
Variables will show up in Material Editor. They're defined like this.
A list of attributes you can expose can be seen below
Data Type | Attribute Function |
---|---|
bool | BoolAttribute |
int/uint | IntAttribute |
float | FloatAttribute |
float2 | Float2Attribute |
float3 | Float3Attribute |
float4 | Float4Attribute |
float/float2/float3/float4 | TextureAttribute |
UiType
Describes how the variable should be represented in the editor.
Name | Description |
---|---|
VectorText | Text boxes and sliders (this is the default) |
Slider | A slider type. Usually combined with a Range setting. |
Color | A Color picker, works on float3 or float4. |
Texture | A texture picker |
CheckBox | On or off, generally used on bool |
UiStep
UiStep
lets you set the amount of each interval or step that the value increases or decreases by.
Default
The Default
lets you specify the default value for the variable. If you're filling a float2
, it should be Default2
, if you're filling a float4
it should be Default4
etc.
Range
The Range
lets you cap the variable to a specific range. If you're filling a float2
, it should be Range2
, if you're filling a float4
it should be Range4
etc.
UiGroup
The UiGroup
is used to sort the variable into a group, subgroup and define its order. So for example, given this..
You end up with this
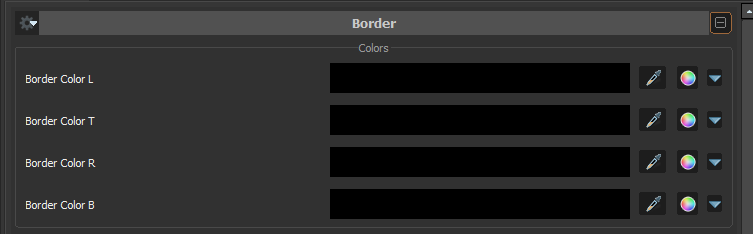
The format is:
Expression
Expression
is used to evaluate an expression on the variable, using the Dynamic Expressions language. A good reason for their use is to save GPU instructions, since they get evaluated on the CPU prior to rendering.
The expression can refer to: the variable itself with this
, as well as to any other variables or features.