Custom ModelDoc nodes
This page describes how you can create custom ModelDoc nodes. This is useful for any gamemode-specific data you may want to attach to specific models.
There are currently 2 types of custom ModelDoc nodes you can create: Generic Game Data and Break Commands.
Generic Game Data
Generic Game Data nodes are just that - a node that can carry arbitrary data on the model and then retrieved in code when needed.
Here's the process for registering and using a custom game data node:
- Define a struct or class. Add the
ModelDoc.GameData
attribute.
Let the gamemode (re)compile. This will make the new nodes appear in ModelDoc.
Assign the node in ModelDoc to the
GameDataList
parent node, or use the node wizard if the node is not present. If you wish to know more, you can read this guide on importing a model.
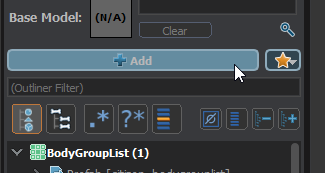
Compile the model.
Now you can use this data in code like so.
Break Commands
Break commands are a more specific alternative to GenericGameData which allows code to be ran when an entity with a model that has a given node breaks via the Breakables class, such as doors, physics props and others.
They work identically to GenericGameData but the class markup looks a little different:
The OnBreak
callback will be called automatically when an entity breaks and has a model with (in this case) break_create_particle
break command.
Other things you can do with this
There are multiple attributes that you can use in order to make your own custom designers/helpers for your custom nodes:
ModelDoc.Axis
ModelDoc.Box
ModelDoc.Sphere
ModelDoc.Capsule
ModelDoc.Cylinder
ModelDoc.Line
ModelDoc.HandPose
ModelDoc.EditorWidget