Triggers
What is a trigger
When a collider component is marked as a trigger, other physics objects pass right through and can detect when they enter or exit the collider.
Detecting Triggers
To detect when an object enters or exits another collider, both GameObjects must have a collider component attached(box, sphere, etc), with the Is Trigger
box checked on one of them.
For a component to detect triggers, it must be on the same GameObject
as one of the collider components.
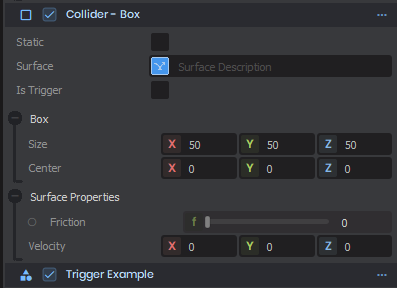
First inherit from Component, Component.ITriggerListener
, then implement public void OnTriggerEnter()
or public void OnTriggerExit
, public void
is necessary. In the parenthesis, add Collider other
to get the entered / exited collider component or GameObject other
to get the GameObject it is on.
Alternatively, with RigidBody.Touching we can get a list of all colliders intersecting us