A Razor Overview
What are Razor components?
Asp.net has this thing called Razor.
Filename is FriendName.razor
As you can see, it's html with c# mixed in. This code creates a new panel class called FriendName
, which just shows a friend's name.
Switching between code and html
If you start something with @, it'll be c#. This is easier to understand in visual studio because you'll get intellisense around it.
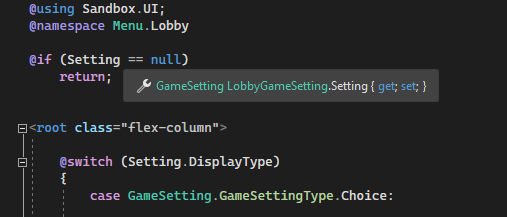
You'll switch back to html as soon as you do a html element, or close the curly braces.
Changing State
The UI will be rebuilt when we think the state has changed (interactions usually). If you change the state and need to force the UI to rebuild, you can call StateHasChanged()
.
This won't rebuild the page instantly, it'll just invalidate so it'll be rebuilt on the next frame - you don't have to worry about calling it multiple times.
Loops
Where Categories
is a list of categories.
Panels
If an html element starts with a capital letter then we assume it's a panel and will try to create/use that class directly. This gives your code a rigidity - because if that panel type changes, your old code will need to too.
You can also set properties on the class directly with attributes. If your attribute starts with a capital letter it will be assumed to be a member of the panel.
Here's LobbyChatEntry
and here's a snippet of LobbyChat
You can see how we use LobbyChatEntry directly, and set Entry directly.
Root element
If your element starts with a <root> element, it'll represent the root of the panel.
The above will end up with a single panel of type Warning
with the class of warning
.
The above will end up with a panel of type Warning
, with a child Panel
with the class warning
Events
You can hook regular panel events with attributes starting with @on
. Here are some examples.
Callbacks
If you're creating a Panel type and it has an event, you can set that action like any other panel attribute.
@ref
You can get a reference to a created panel using @ref. References are only available after the UI has been built (because the UI elements won't exist before then). After the ui is built OnAfterTreeRender
is called - which you can override to access the references.
It should be noted that the reference may change when the UI gets re-built due to changes. If you're accessing panels via ref then this is something you should be thinking about and handling.
For example, in the code below ThePanel
will be null until showPanel is true and it is rebuilt. If you set showPanel to false again, ThePanel
will become null again after a rebuild.
Early Return
You can return early in your component if you don't want to render it for some reason..
@code
The @code
block allows you to add code which isn't part of the UI. Here you can override any member that you would usually be able to override on Panel.
@code
tag does have some limitations:
- Visual studio code analysis and code cleanup do not run.
- C# Codegen is not run on .razor embedded code right now
The created Panel
class is declared as partial
, so you can also add "code behind" by creating a .cs file with a partial class if you choose.
There are some special Razor specific methods that you can override, which will make things a bit easier.
OnParametersSetAsync OnParametersSet
This is called when any of the Parameters change. This allows you an opportunity to reinitialize any other data.
The Async version is useful if you have to query some other data source based on external input.
virtual void OnAfterTreeRender( bool firstTime )
Called after the UI has been built. At this point all the child panels have been created, so if you have created anything with @ref
that you need to initialize in some other way, this is the best place for that.
int BuildHash()
We need to know when to rebuild the UI. We only want to do it when the state has changed - because it would be a huge waste of time to do it any other time.
By default the UI is updated after any event (mouse over, click etc) or when a parameter changes.
If you want to kind of watch some external variables and update when they change, you can use the BuildHash function for that.
In the example above, we're showing the UI in the component so want to update it every time it changes. We do this by providing the hash of the string we're using in the hash.
In the example above we rebuild the UI whenever the player's lobby and ingame status changes.
<style>
You can embed styles in your component. The styles will only apply to your component (and its children).
Stylesheets
You can include stylesheets with @attribute
Note that when splitting up your C#, Razor and CSS code into different files, you will want to follow the following format: Example.razor
, Example.razor.cs
, Example.razor.scss
. This will fold all files into one in Visual Studio as well as ensure that live updates to your CSS are recognised. Also, if you name everything the same, you can just write @attribute [StyleSheet]
.
Two Way Binds
Sometimes you want to bind a variable to a control, and if it changes it, sync the value back. That's what two way binds are.
You create a two way bind using :bind
after the attribute name.
In the example above, the value is initially set to 32. If the value changes in the Slider, then it sets our IntValue to that value. If our value changes, it sets the Slider value.