Attributes and Component Properties
Attributes
Attributes in C#, generally, are metadata added to code elements such as classes, methods, or properties to assign additional semantic information that can be accessed at runtime or compile time.
In S&box, we have a few attributes that do special things in the Scene Editor.
Firstly, if you put [Icon]
, [Category]
, and [Title]
before defining your Component class, you can give your Component an icon, category, and custom title!
The [Title]
Attribute is primarily useful if the title of your component ought to be different than the auto-set title being your Component's class name.
An example of this (from the default Box Collider component) would be:
You can find a list of Icons on Google's Material Design Icons github..
Component Properties and You
When you're creating a new Component, you may want to create variables or other objects that are exposed in the Inspector so they can be changed manually in the editor (while editing or while playing), or so you can refer to specific GameObjects or Components in the scene without getting them through code.
You can do this by adding [Property]
before defining the object. You can also add other Attributes in addition to Property, to augment the Property in the editor.
For example, take a look at this Property from the CitizenAnimationHelper component:
This creates a new public float variable called Height with an auto-implemented Getter and Setter (properties need to have a Getter and Setter or they won't work) that will display in the editor with a slider that can be set between 0.5 and 1.5, with the title 'Avatar Height Scale'.

You can then drag those components into the inspector and your code will use the specific instance of that dragged-in component when executing the code that references that property!
You can also place [Property] on more than just primitive types like floats and integers. GameObjects, components like CharacterController
and components/classes you create yourself also work.
See the end of the article for some more examples.
Reference
Range
As previously discussed, you can set valid ranges for Properties which will give them a slider instead of a text entry. The precision of the slider is based on what kind of variable you set-- an integer will go increments of one, a float will have decimals.
Group
Groups of properties. These will appear under the same group in the inspector to make the inspector look cleaner.
ToggleGroup
Groups with a bool that you can toggle on/off in the inspector. To be clear- you are toggling on/off the bool, you need to do something within the code to make it respect the toggling, or the bool will just be turning on and off.
TextArea
[TextArea]
allows you to give a [Property]
that takes text a larger text field, if you wanted to use it for a whole paragraph or more instead of a small string. Just add it alongside an existing [Property]
attribute.
Enums
Enums are special, each underlying enum member can have [Icon]
and [Description]
and [Title]
attributes when being defined, and then when you make the Property refer to the enum type you created with those definitions, it will show up in the editor as a selector between your different enums with the fancy descriptions and titles and such for each one. Nice!
Result:
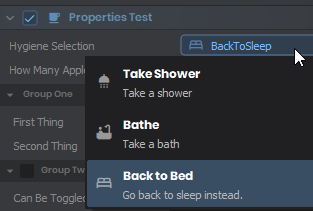
Lists
Lists can be properties just like any other variable, and they work like you'd expect.
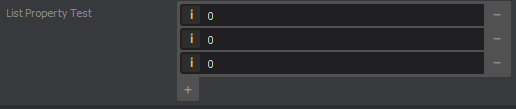
Other objects that can be used with [Property] in components
Pretty much anything you'd expect to work can be used as a Property in a Component. Here are some other examples:
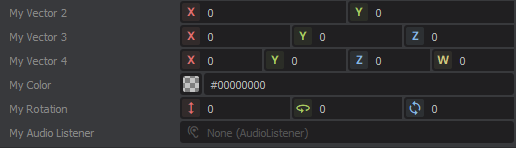
Display Info
The DisplayInfo library can be used to define or display information about type and type members.
Properties
The following table lists the properties of DisplayInfo, and what attributes are related to each property. In the case that multiple attributes are listed, they are in order of precedence.
Property | Attribute | Target |
---|---|---|
ClassName | Both | |
Namespace | Both | |
Fullname | Both | |
Name | TitleAttribute, DisplayAttribute | Both |
Description | DescriptionAttribute, DisplayAttribute | Both |
Group | CategoryAttribute, DisplayAttribute | Both |
Icon | IconAttribute | Both |
Order | OrderAttribute, DisplayAttribute | Member |
Browsable | BrowsableAttribute | Member |
Placeholder | PlaceholderAttribute | Member |
Alias | AliasAttribute | Both |
Tags | TagAttribute | Both |
Defining info for DisplayInfo
To define information for the DisplayInfo library, use the attributes listed in the table above. For example, this Item
class defines the Name and Icon.
This class will have its Name
as Item and Icon
as luggage.
Fetching info from DisplayInfo
To get the DisplayInfo of a type or member, use the static For
, ForType
, or ForMember
methods of Sandbox.DisplayInfo
. Then, the information can be accessed as properties of the DisplayInfo. Here's an example in a class of the name 'Player Pawn':