Widget Docking
Widget Docking
Widget docking makes it easy for users to layout the UI you make how they want. The editor makes heavy use of docking to allow for these customizations. If you just want to add a widget to an existing dock window (such as the editor or hammer), read this.
The DockWindow widget provides a DockManager for creating docks and adding widgets to them.
The DockWindow widget provides a DockManager for creating docks and adding widgets to them.
To get started, create a class the inherits the DockWindow widget and add some method of opening it. If you're not sure how to open it, read this.
Create a widget, and add the widget to the dock manager in your constructor like this
The result of adding these two lines will look something like this
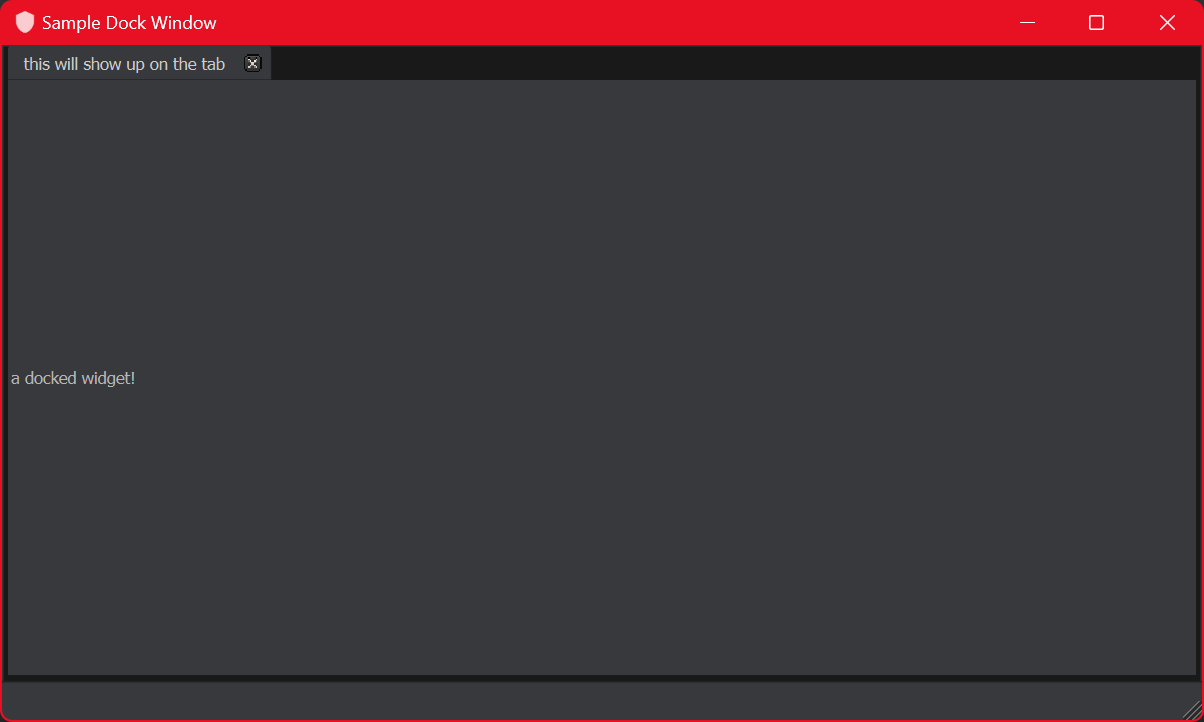
Duplicate the code above, and you will have two docks each with a widget of their own
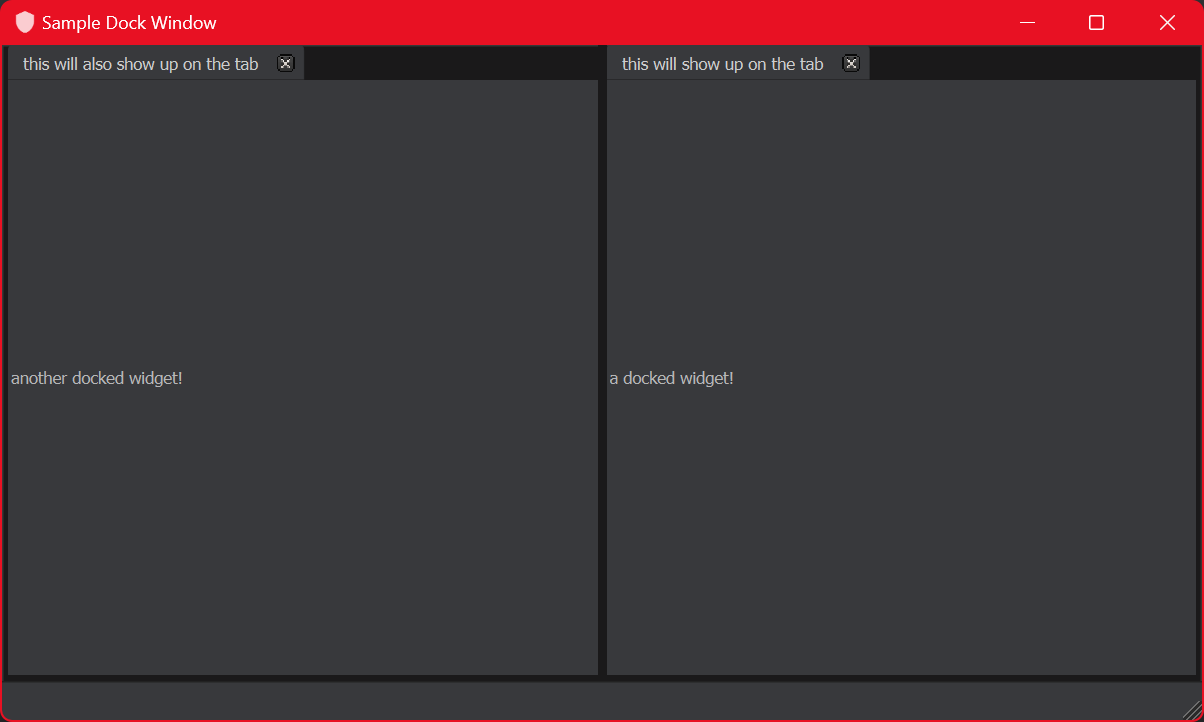
With just two docks, the widgets can now be dragged around inside the dock window and oriented how the user desires
You can have multiple widgets docked ontop each other by using DockArea.Inside as the third parameter of DockManager.AddDock
You can have multiple widgets docked ontop each other by using DockArea.Inside as the third parameter of DockManager.AddDock
You can choose what widget to dock ontop of by specifying the first parameter
Which will look like this
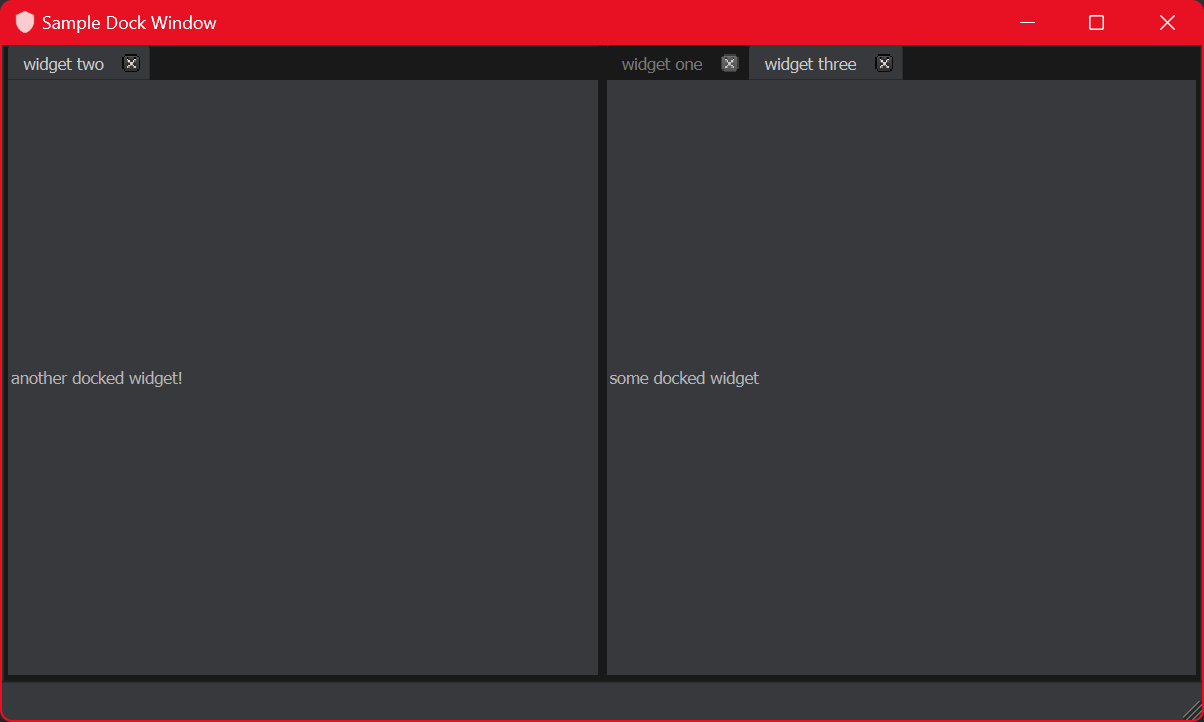
Dock Properties
Remember that these widgets can be move around however the user wants. If you want to add restrictions, you can use a DockProperty eg. If you want to add restrictions, you can use a DockProperty eg.
This will prevent the widget from being docked elsewhere. The widget can still be closed, so to prevent that you can add the HideCloseButton property.
Registering Docks
You may want to let players close and reopen docked widgets. You can do this by registering a dock and creating a widget with the the same name as the dock.
We will use a view menu in the menu bar to list the widgets that can be hidden. This will let users hide and show the widget.
Add a method in your dock window's class for when the view menu opens
Then add the view menu to the menu bar in your dock window's constructor
It should appear like this
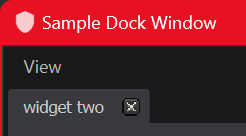
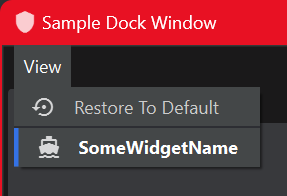
Clicking the option in the view menu will now hide and show the widget