UI Tips & Tricks
There's 2 ways to make UI right now in S&box. You can either create Panels entirely through code, or you can use Razor to create panels with HTML and CSS that can also use C#.
It is recommended to use Razor.
Tips and Tricks
Steam Avatars
To display a Steam avatar, specify the source using avatar:SteamId
, like this:⤶
You can adjust the avatar size using SCSS classes to style it as needed.⤶
Panel Classes
- You can set Panel classes - See Panel.SetClass, and Panel.BindClass
Debugging
- If a panel is not behaving as expected you can troubleshoot it using the 'UI Panels' inspector. If its not enabled, you can do so under View > UI Panels.
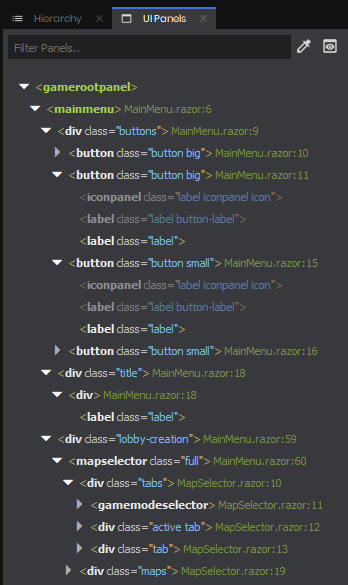
Drawing Polygons
Example:
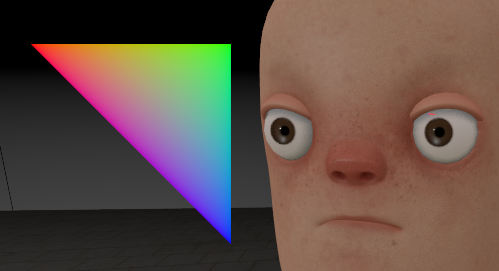