Creating a Tool
What is a Tool?
Sometimes your game may require certain tools that s&box may not provide to you out of the box. In cases like these it can be beneficial to develop your own set of tools that allow you to automate and/or ease certain tasks.
A tool can be anything from an editor with a few sliders and a text entry to a turntable spritesheet renderer.
If you want others to be able to use your tool, the convention is to upload it to GitHub with the sbox and sbox-tool topics on the repository.
If you prefer to learn from example, you may want to look at these repositories.
Creating a new Tool
If you are making a game-specific tool, then all you have to do is create a new folder named editor
in the code folder of your game project. From here you can follow the next section and place any .cs files for your tool in here. This will ensure that the tool is only loaded when you are editing that game project.
If you are not making a game-specific tool, and instead want to make a tool that is always loaded in the editor no matter what, you must create a new "Tool" project.
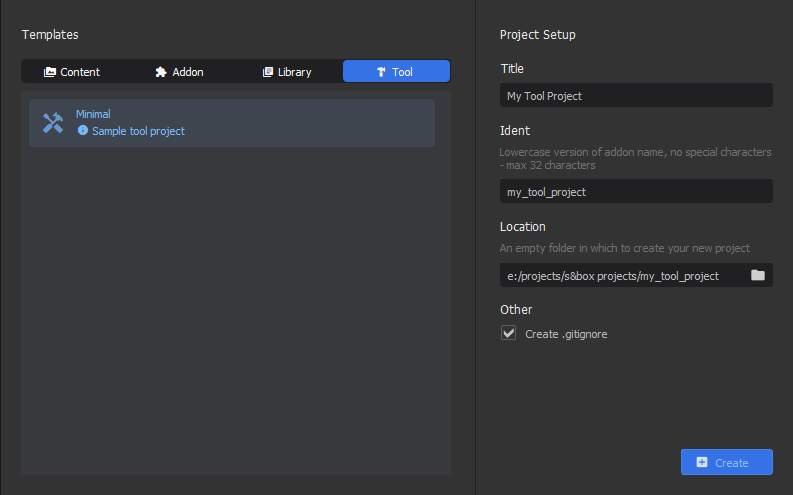
Defining a Tool Window
When your tool is loaded in the editor, it will look for any DockWindow
that has a [Tool]
attribute and add it to the Tools Menu and sidebar in your Editor. You can then open that window at any time by clicking on the tool in the list.
You can define a custom window of your own like such:
Defining a Tool Dock
A Dock is similar to a Window but is meant to be primarily used while docked (but can still be pulled away as a floaty window). Docks are added to the View Menu in your Editor and can be toggled on/off.
You can define a custom dock like this:
Learn more about the dock attribute here
Defining a Tool Menu
A Menu is a custom drop-down added to your Editor's Menu Bar. These are useful for holding shortcuts and options that are specific to your tool that you would want regular and/or easy access to.
You can create one like such: