Material API
What is the Material API
The Material API is a collection of helper methods & texture inputs to describe the surface of your material. The main purpose of the Material API is to prepare your data and pass it to a ShadingModel.
The Material API is automatically used when you add the common/pixel.hlsl
include to your pixel shader.
Texture Inputs
By default, the Material API provides a set of texture inputs. These texture inputs can be used to automatically populate the Material struct for you. To populate your Material object, simply call GatherMaterial
using your PixelInput
as the argument. GatherMaterial will automatically transform your normal map to be within object space, set up texture compression & pack different textures into one texture to reduce memory.
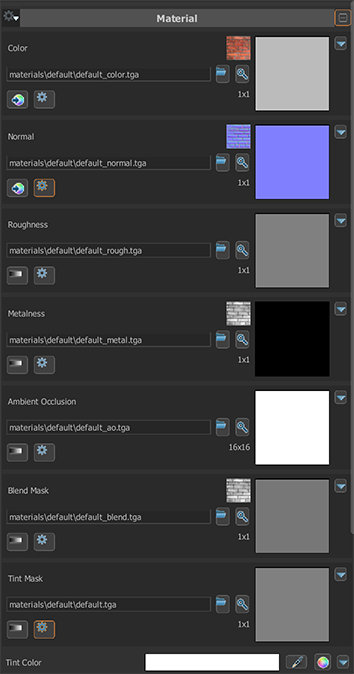
Material
The following is an overview of the Material struct defined in common/pixel.material.structs.hlsl
. It is included automatically by common/pixel.hlsl
.
Property | Type | Default Value | Notes |
---|---|---|---|
Albedo | float3 | (1.0, 1.0, 1.0) | |
Emission | float3 | (0.0, 0.0, 0.0) | |
Opacity | float | 1.0 | |
TintMask | float | 1.0 | |
Normal | float3 | (0.0, 0.0, 1.0) | The normal vector of the material in world space |
Roughness | float | 1.0 | |
Metalness | float | 0.0 | |
AmbientOcclusion | float3 | (1.0, 1.0, 1.0) | Can be normal AO or bent normal AO |
Sheen | float3 | (0.0, 0.0, 0.0) | |
SheenRoughness | float | 0.0 | |
Clearcoat | float | 0.0 | |
ClearcoatRoughness | float | 0.03 | |
ClearcoatNormal | float3 | (0.0, 0.0, 1.0) | |
Anisotropy | float | 0.0 | |
AnisotropyRotation | float3 | (1.0, 0.0, 0.0) | |
Thickness | float | 0.5 | |
SubsurfacePower | float | 12.234 | |
SheenColor | float3 | sqrt(baseColor) | |
SubsurfaceColor | float3 | (0.0, 0.0, 0.0) | |
Transmission | float3 | (1.0, 1.0, 1.0) | |
Absorption | float3 | (0.0, 0.0, 0.0) | |
IndexOfRefraction | float | 1.5 | |
MicroThickness | float | 0.0 |
Shading Models
Different shading models may use different subsets of the properties available in the Material struct.
Albedo
, Emission
, Opacity
, TintMask
, Normal
, Roughness
, Metalness
, and AmbientOcclusion
are available across all shading models.
Sheen
, SheenRoughness
, Clearcoat
, ClearcoatRoughness
, ClearcoatNormal
, Anisotropy
, AnisotropyRotation
are unused by the standard shading model at the time of writing.
Shading Model | Thickness | Subsurface Power | Sheen Color | Subsurface Color | Transmission | Absorption | Index of Refraction | Micro Thickness |
---|---|---|---|---|---|---|---|---|
Standard | ❌ | ❌ | ❌ | ❌ | ❌ | ❌ | ❌ | ❌ |
Subsurface | ✅ | ✅ | ❌ | ✅ | ❌ | ❌ | ❌ | ❌ |
Cloth | ❌ | ❌ | ✅ | ❌ | ❌ | ❌ | ❌ | ❌ |
Refraction | ❌ | ❌ | ❌ | ❌ | ✅ | ✅ | ✅ | ✅ |