Traces
What are traces
Traces are imaginary lines. When you run a Trace you get a TraceResult - which tells you what the line hit.
So for example, if you, as the player, wanted to spawn a box. You'd run a Trace from the player's eyeball to 200 units in the direction that the player is looking. The TraceResult would show that it hit a point and now you know where to place the box.
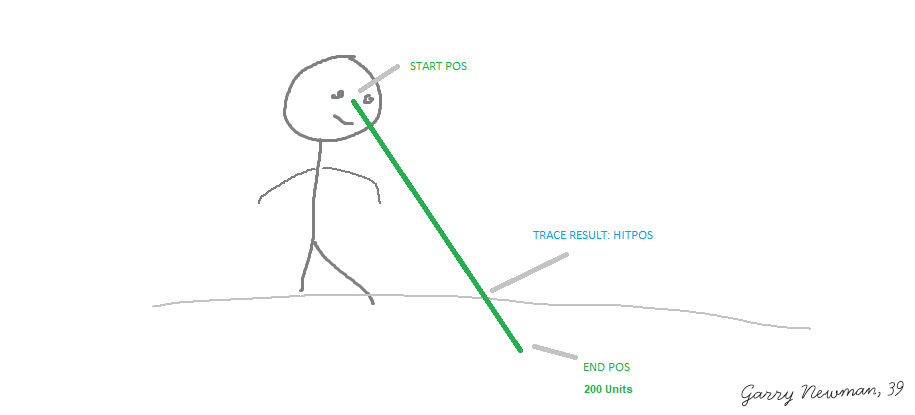
Tracing
Traces are constructed using the Trace static functions, and configured using member methods. You run the trace and get the result using Run().
All configuration methods return a new Trace
object. This is a convenience thing (known as builder pattern), so you can format traces like this:
or like this:
Size
In the example above the Size()
call describes the size of an AABB to trace.
This means instead of tracing a simple line, you're tracing a bigger cube along the line.
Please note that this cube would be axis aligned, meaning it cannot "rotate".
RunAll
Instead of ending your Trace with Run()
function, you can use the RunAll()
function.
Using RunAll()
will tell your Trace that you don't want to stop at the first entity who's been hit. Instead it'll return an array containing a TraceResult for each entity that was hit along the path of the trace.
An example use case for this function would be for bullets that penetrate through objects.
TraceResult
The Trace Result is a simple structure. It gives you information such as whether and where the Trace hit, what it hit, etc.
Here you can see testing whether the trace hit and using the hit position to spawn an entity 10 units above it.
Box, Sphere, Capsule, PhysicsBody traces
In addition to ray/line Traces, you can also trace with other shapes, using other static methods of the Trace
class, for example an Axis-Aligned Bounding Box trace:
Note how the box shape does NOT rotate with the player's view.
The hit position will always be in the center of a given shape. Other shapes, such as spheres and capsules, work in the exact same way.
Trace.Body
allows you to trace any PhysicsBody
, and therefore any custom shape. It DOES preserve the body's rotation.