Custom Asset Types
Custom assets are things you can define yourself. They give you a nice inspector window and they're hotloaded in-game, which means you can whip things up pretty quickly if you're using them.
You can find plenty of examples of assets throughout s&box, here's a snippet from the clothing asset:
It is important to note, you should ensure that your filetype is all lowercase, otherwise it will fail to register.
[GameResource("Clothing Definition", "clothing", "..." )]
defines the title (Clothing Definition
), file extension (.clothing
), and a description of the asset type- You should ensure that your filetype is all lowercase, otherwise it will fail to register/save.
- Note that these are just JSON files with pretty faces and file types easy for the game to locate and developers to work with
Attributes
S&Box utilizes an attribute system to make custom assets easier to manipulate. Any of these attributes can be used before the properties declaration that you want it to affect (See the above example.) For a full list, use the API reference
[ResourceType()]
Supports specifying the file extension of a resource you want to use, which will add a navigator to the inspector.
Examples:
[ResourceType( "png" )]
[ResourceType( "vmdl" )]
.
In addition, it also supports asset files, including custom ones. e.g. [ResourceType( "sound" )]
or [ResourceType( "clothing" )]
.
[Category()]
Allows properties to be grouped in with properties of similar interest in a collapsible block in the editor. Say we have a GameResource for custom props we can do this: [Category("Prop Info")] public string Name {get; set;}
[HideInEditor]
Used for backend properties that should not be edited in the editor.
[Description()]
Used to provide a brief description of the property being edited in the editor. We can add this to the above Name property like this: [Description("Name shown when spawning prop")]
[JsonIgnore]
This attribute will prevent the value of this property from being serialized to the json file being created. This means the property will not be saved and loaded through the asset file. Good usage of this is when you have helper properties in your GameResource type, these properties wouldn't need to be saved persistently since they aren't data fields.
Example: [JsonIgnore] public int TotalWeight => Weight * Quantity;
Using the inspector
Now that you have everything set up, you can use the inspector tool to create assets of your custom type.
Go to the "Assets Browser" tab in the s&box editor.
Right click a folder in your addon and click "New <Your Asset Name>". Typically you'd want to put these in some sort of
data
folder.
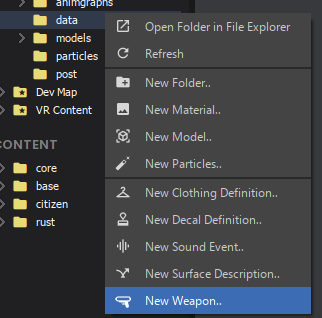
- Enter a name for your asset
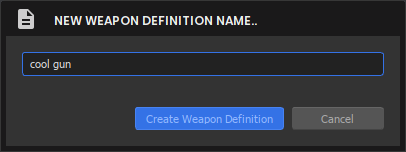
Go to the "Inspector" tab and set your asset up how you want it.
Click the save button (looks like a floppy disk) to save your asset.
Accessing assets
All assets are loaded when you first start the game, there are several ways you can access them:
ResourceLibrary.Get<T>
When assets are loaded they are stored in a dictionary with their path, you can access these with ResourceLibrary.Get<T>
.
ResourceLibrary.TryGet<T>
TryGet<T>
serves a similar purpose to Get<T>
but returns a bool indicating whether the resource could be found. If the resource was found, the method provides it through an out
parameter. This is just a slightly cleaner way to handle missing assets.
PostLoad
When assets are loaded they call their PostLoad method, you can use this to store a list of your assets for later use.
Possible solution for Asset.LoadResource returning null
Make sure the class inheriting GameResource has an anonymous constructor, eg.