CSharp Basics
New to C#? No clue what this image means? Lets fix that!
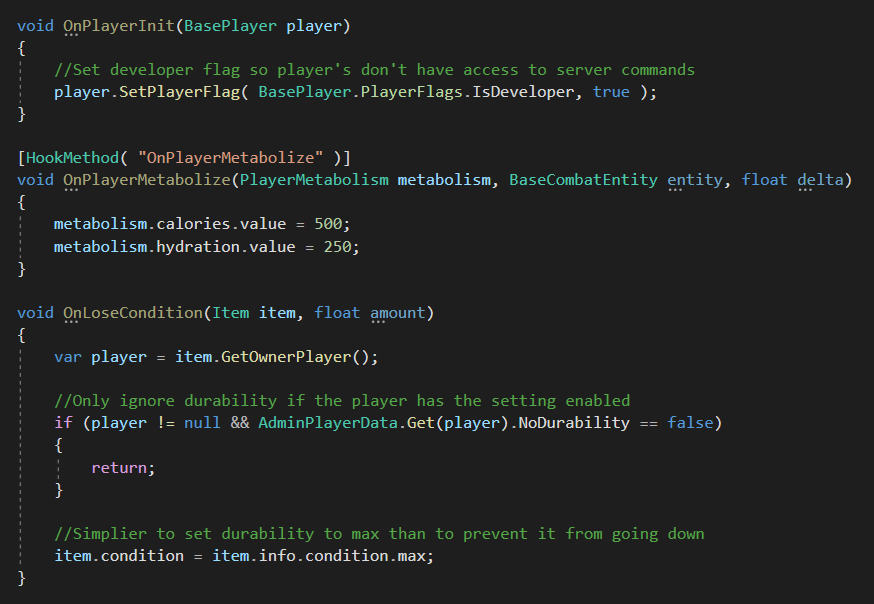
Labeled
Each section below will explain a part of this image.
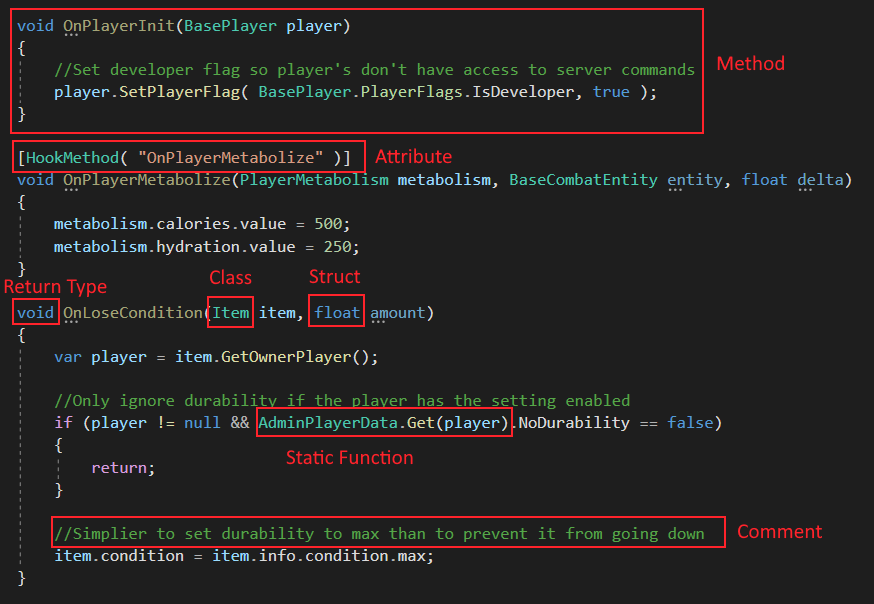
Comments
What is all that green text scattered around?
Comments are not counted as code and exist purely to explain the purpose or function of code around it.
Comments are often written directly above the code they explain, so look right below the green text comments to see what they are explaining.
They are written by putting two forward slashes //
at the start of a line. Anything after those //
will be treated as comments and ignored by the compiler.
Compiler
Hang on, what the heck is a compiler?
A compiler simply takes your code (a text file) and turns it into an executable program that can run on computers.
For modding Rust there is a special compiler that can take your code, compile it into a program and run it on the fly without needing to restart or pause the server.
Functions / Methods
Can be referred to by either name.
Functions let us group our code into blocks. This lets use the same code in multiple places and organize by it's purpose.
They can be executed by writing the name of the function with two parenthesis ()
after it.
For the examples below, the function Hook()
represents a function the modding framework knows about and runs.
What Is Void
Why do you need to put void before every function!
In CSharp we use void
to indicate we want the function to return nothing.
It is designed this way to help the compiler find the start of the function.
If we want it to return something else we put the Type
in front of the function.
But what is a Type?
Types
One of the major features of CSharp but present in most languages.
A Type is a grouped block of code and variables.
This allows you to re-use code for multiple things and keep it organized by it's purpose.
However, there are two different kinds of types:
Class
The main way you will use types
to structure and group your code is with classes
.
They can easily be identified by their Cyan color when using Visual Studio
.
Examples:
BaseEntity
BasePlayer
StorageContainer
Monobehaviour
Coroutine
Classes are never stored directly: the actual data is stored somewhere in RAM and your variable stores the location of the class's data.
Null
When using classes, null
represents 'nothing' and is the default value when a class has not been assigned any data.
Struct
You will use them a lot, however it is rare you will create your own struct
.
Examples:
int
float
long
Vector3
Quaternion
string
A struct
will still let you group code and variables together. The main difference is how the data is stored.
As a beginner, you can think of a struct as the same as a class.
Later on read the section on Classes and Structs Explained to gain a better understanding.
Return Types
Back to functions: let's say we want to add together numbers and return the result as a new number.
In this case, we will put int
in front of the function: which represents a whole number.
This will tell the compiler that we want to have a new function and it has to return a number.
Parameters
Can also be referenced as arguments
Parameters are data or options passed into a function / method.
Lets say we want to make a function to count the amount of letters in a player's name