Revision Difference
SavedGames#560520
<cat>Code.Misc</cat>⤶
<title>Saved Games</title>⤶
⤶
# Saved Games⤶
⤶
You can enable support for Saved Games in the Project Settings for your game.⤶
⤶
⤶
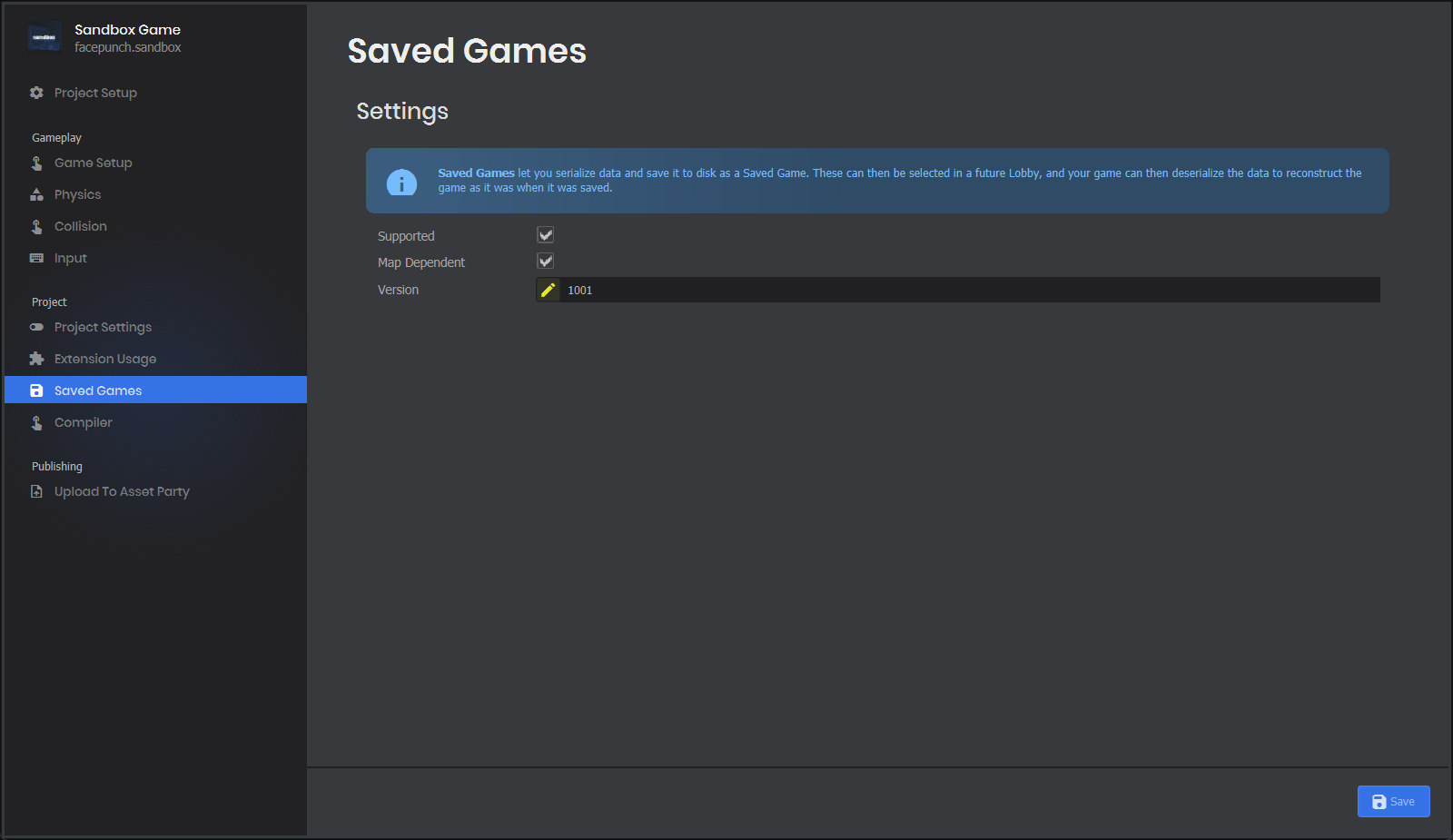⤶
⤶
### Supported⤶
⤶
Whether or not Saved Games are supported.⤶
⤶
### Map Dependent⤶
⤶
Whether or not Saved Games are saved per-map or if Saved Games should work across any map.⤶
⤶
### Version⤶
⤶
You can increase this value to mark any Saved Games with an older version as being incompatible. Useful if you change the format of your Saved Games in the future.⤶
⤶
# Saving Games⤶
⤶
You can serialize your Saved Games in any way you want to. You could use Json, or you could use a BinaryWriter for example.⤶
⤶
## API⤶
⤶
The API for Saved Games is very simple. You can create a new `SavedGame` object, which you can use to set metadata about the Saved Game such as the name or the map. You can then either set raw data or use Json for serialization.⤶
⤶
## Using Json⤶
⤶
Here is a crude example of how you can serialize data using Json for a Saved Game.⤶
⤶
```csharp⤶
public class MyPropState⤶
{⤶
public string Model { get; set; }⤶
public Vector3 Position { get; set; }⤶
public Rotation Rotation { get; set; }⤶
}⤶
⤶
public class MySavedGameState⤶
{⤶
public List<MyPropState> Props { get; set; }⤶
}⤶
⤶
var save = new MySavedGameState();⤶
save.Props = new List<MyPropState>();⤶
⤶
foreach ( var m in All.OfType<Prop>() )⤶
{⤶
if ( !m.IsFromMap )⤶
{⤶
var data = new MyPropState();⤶
data.Model = m.GetModelName();⤶
data.Position = m.Position;⤶
data.Rotation = m.Rotation;⤶
save.Props.Add( data );⤶
}⤶
}⤶
⤶
var s = new SavedGame();⤶
s.Name = "Test Save";⤶
s.SetData( JsonSerializer.Serialize( save ) );⤶
Game.Save( s );⤶
```⤶
⤶
## Using BinaryWriter⤶
⤶
```csharp⤶
using var s = new MemoryStream();⤶
using var w = new BinaryWriter( s );⤶
⤶
var allProps = All.OfType<Prop>().Where( p => !p.IsFromMap );⤶
⤶
w.Write( allProps.Count() );⤶
⤶
foreach ( var p in allProps )⤶
{⤶
w.Write( p.GetModelName() );⤶
w.Write( p.Position );⤶
w.Write( p.Rotation );⤶
}⤶
⤶
var save = new SavedGame();⤶
save.Name = "Test Save";⤶
save.Data = s.ToArray();⤶
Game.Save( save );⤶
```⤶
⤶
# Loading Games⤶
⤶
When a game has been started from a previously Saved Game, the `LoadSavedGame` method will be called on your `GameManager` class. You can use this to deserialize the Saved Game data and restore your game's state appropriately.⤶
⤶
## Using Json⤶
⤶
Following the example above for saving games with Json, you could use the following to deserialize the Saved Game.⤶
⤶
```csharp⤶
public override void LoadSavedGame( SavedGame save )⤶
{⤶
var jsonString = save.GetDataAsString();⤶
var deserialized = Json.Deserialize<MySavedGameState>( jsonString );⤶
⤶
foreach ( var d in deserialized.Props )⤶
{⤶
var prop = new Prop();⤶
prop.SetModel( d.Model );⤶
prop.Position = d.Position;⤶
prop.Rotation = d.Rotation;⤶
}⤶
}⤶
```⤶
⤶
## Using BinaryReader⤶
⤶
Following the example above for saving games using a BinaryWriter, you could use the following to deserialize the Saved Game.⤶
⤶
```csharp⤶
public override void LoadSavedGame( SavedGame save )⤶
{⤶
using var s = new MemoryStream( save.Data );⤶
using var r = new BinaryReader( s );⤶
⤶
var propCount = r.ReadInt32();⤶
⤶
for ( var i = 0; i < propCount; i++ )⤶
{⤶
var prop = new Prop();⤶
prop.SetModel( r.ReadString() );⤶
prop.Position = r.ReadVector3();⤶
prop.Rotation = r.ReadRotation();⤶
}⤶
}⤶
```⤶