math.ease
An easing library that allows you to interpolate with various kinds of smoothing functions. To use with Lerp, input what you would to the fraction argument in one of these easing functions and then the output of that into the Lerp fraction argument.
Example
Example usage with Lerp and math.ease.InSine
Output: 0.076120467488713
0.25
0.38060233744357
1.25
A brief visual example of different easing methods
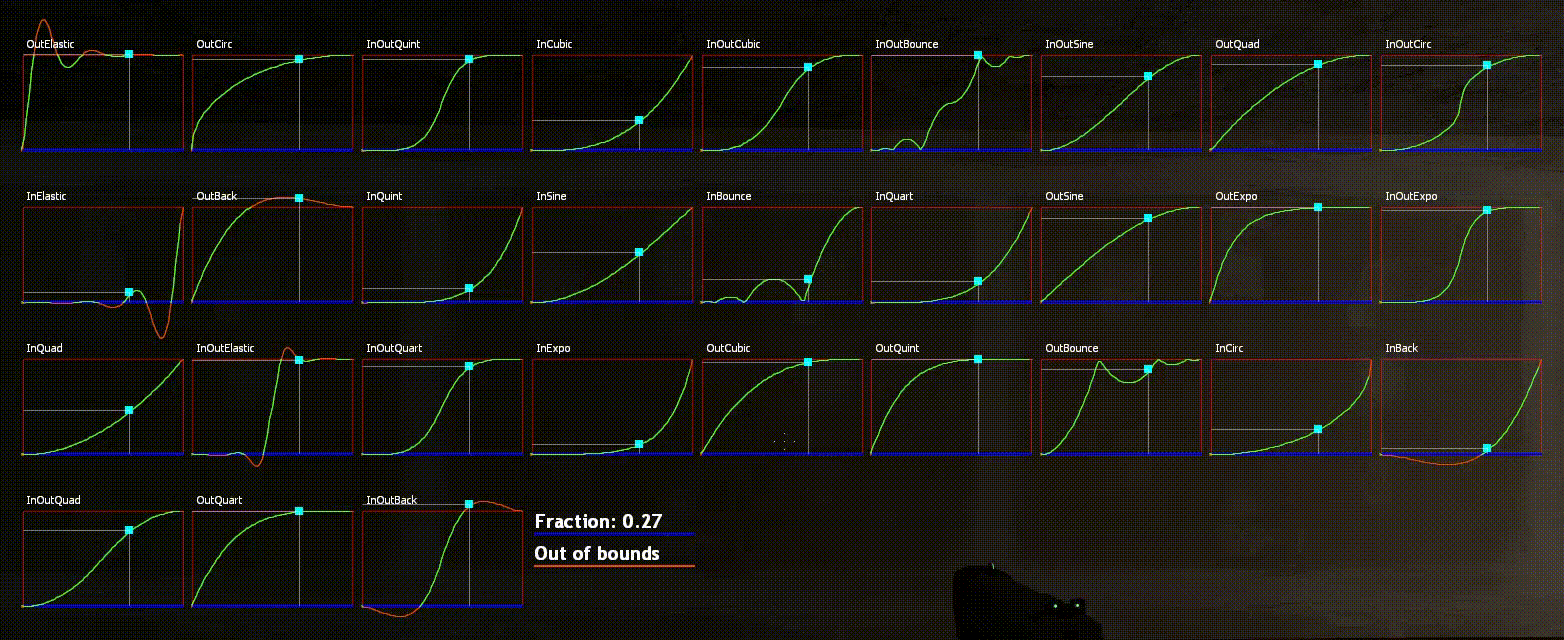
Methods
Eases in by reversing the direction of the ease slightly before returning.
This doesn't work properly when used with Lerp as it clamps the fraction between 0 and 1. Using your own version of Lerp that is unclamped would be necessary instead.
Eases in like a bouncy ball.
This doesn't work properly when used with Lerp as it clamps the fraction between 0 and 1. Using your own version of Lerp that is unclamped would be necessary instead.
Eases in like a rubber band.
This doesn't work properly when used with Lerp as it clamps the fraction between 0 and 1. Using your own version of Lerp that is unclamped would be necessary instead.
Eases in using an exponential equation with a base of 2 and where the fraction is used in the exponent.
Eases in and out by reversing the direction of the ease slightly before returning on both ends.
This doesn't work properly when used with Lerp as it clamps the fraction between 0 and 1. Using your own version of Lerp that is unclamped would be necessary instead.
Eases in and out like a bouncy ball.
This doesn't work properly when used with Lerp as it clamps the fraction between 0 and 1. Using your own version of Lerp that is unclamped would be necessary instead.
Eases in and out by cubing the fraction.
Eases in and out like a rubber band.
This doesn't work properly when used with Lerp as it clamps the fraction between 0 and 1. Using your own version of Lerp that is unclamped would be necessary instead.
Eases in and out using an exponential equation with a base of 2 and where the fraction is used in the exponent.
Eases in and out by raising the fraction to the power of 4.
Eases in and out by raising the fraction to the power of 5.
Eases out by reversing the direction of the ease slightly before finishing.
This doesn't work properly when used with Lerp as it clamps the fraction between 0 and 1. Using your own version of Lerp that is unclamped would be necessary instead.
Eases out like a bouncy ball.
This doesn't work properly when used with Lerp as it clamps the fraction between 0 and 1. Using your own version of Lerp that is unclamped would be necessary instead.
Eases out like a rubber band.
This doesn't work properly when used with Lerp as it clamps the fraction between 0 and 1. Using your own version of Lerp that is unclamped would be necessary instead.
Eases out using an exponential equation with a base of 2 and where the fraction is used in the exponent.