cam.Start3D2D
Description
Sets up the model transformation matrix to draw 2D content in 3D space and pushes it into the stack (cam.PushModelMatrix).
Matrix formula:
This must be closed by cam.End3D2D. If not done so, unexpected issues might arise.
Arguments
2 Angle angles
Angles of the 3D2D context.
+x in the 2d context corresponds to +x of the angle (its forward direction).
+y in the 2d context corresponds to -y of the angle (its right direction).
If (dx, dy) are your desired (+x, +y) unit vectors, the angle you want is dx:AngleEx(dx:Cross(-dy)).
3 number scale
The scale of the render context.
If scale is 1 then 1 pixel in 2D context will equal to 1 unit in 3D context.
Example
Makes a floating rectangle with text above where the player is looking at, pointing at the player
Output: 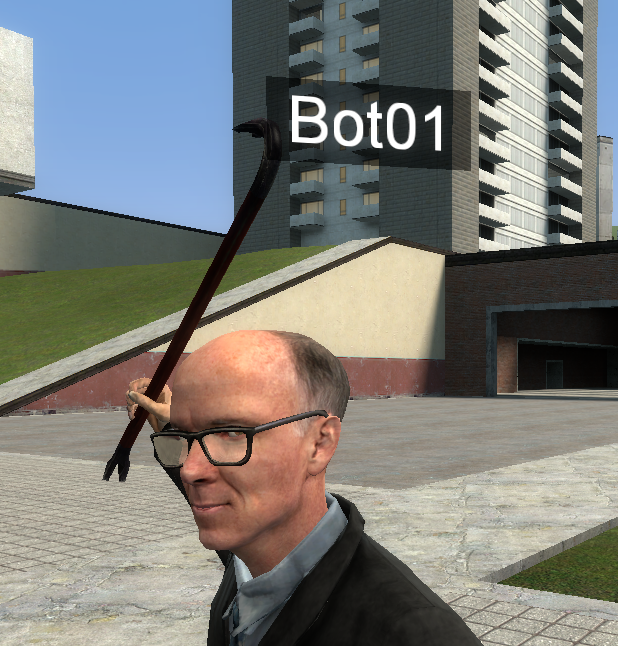
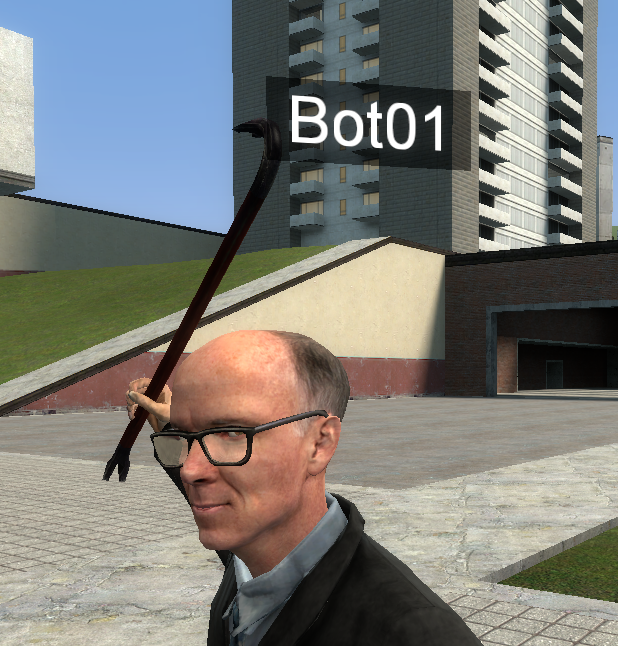
Example
An example on how to correctly rotate and position 3D2D "screen" attached to a prop.
Output: 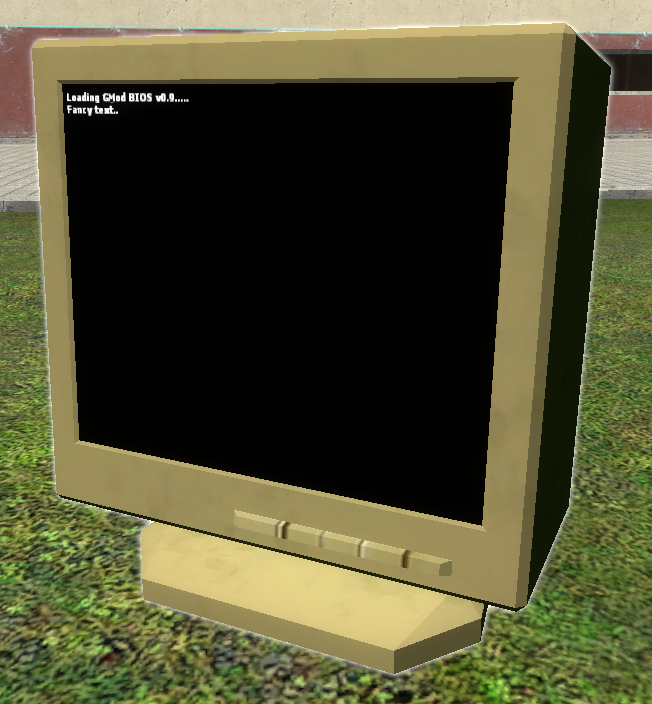
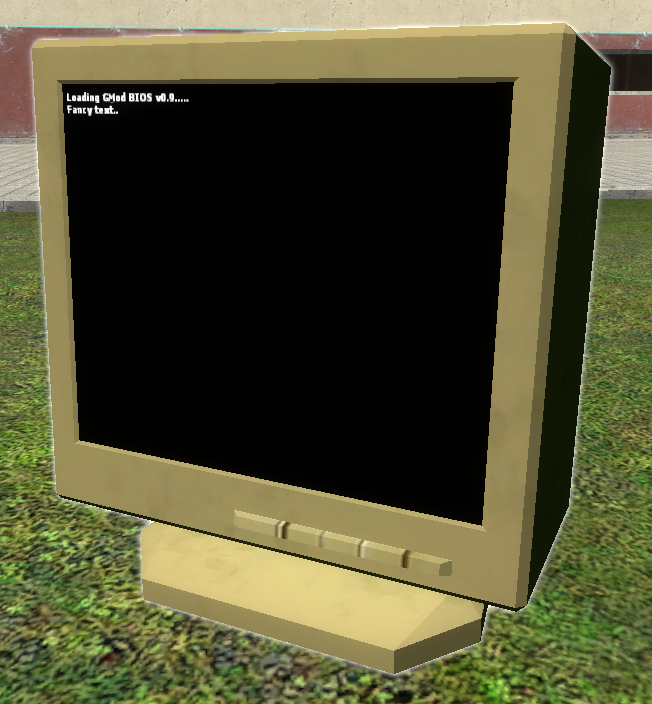