GM:PostPlayerDraw
Description
Called after a player in your PVS (Potential Visibility Set) was drawn.
This is a rendering hook which provides a 3d rendering context.
Arguments
Example
Show each player's name above their model.
Example
Draw a headcrab hat on all players.
Result:
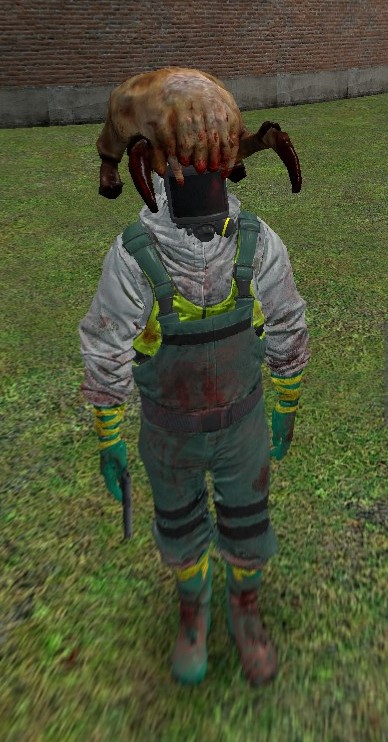
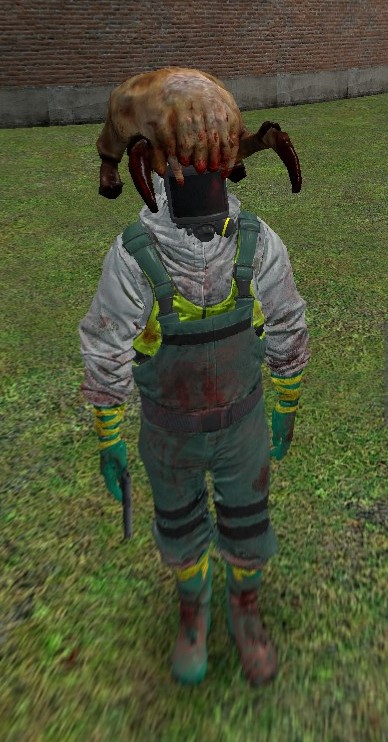