cam.PushModelMatrix
Description
Pushes the specified matrix onto the render matrix stack. Unlike opengl, this will replace the current model matrix.
This does not work with cam.Start3D2D if
multiply
is false.When used in the Paint function of a panel, if you want to rely on the top-left position of the panel, you must use VMatrix:Translate with the (0, 0) position of the panel relative to the screen.
Arguments
2 boolean multiply = false
If set, multiplies given matrix with currently active matrix (cam.GetModelMatrix) before pushing.
Example
Simple function to draw rotated and/or scaled text.
Output:
Example
Rotating a box while keeping it's matrix positions relative to the panel
Output: 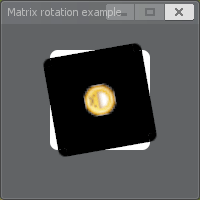
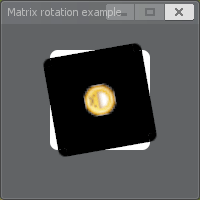