Description
DRGBPicker is an interactive panel which can be used to select a color hue.
See DColorCube for a color picker which controls brightness and saturation.
See DColorMixer for a color picker that allows control over hue, saturation, and brightness at once.
View source
Parent
Derives methods, etc not listed on this page from DPanel.
Events
Function which is called when the cursor is clicked and/or moved on the color picker. Meant to be overridden.
Methods
This is used internally - although you're able to use it you probably shouldn't.
Returns the color at given position on the internal texture.
Returns the color currently set on the color picker.
Sets the color stored in the color picker.
This function is meant to be called internally and will not update the position of the color picker line or call DRGBPicker:OnChange
Example
Creates a color picker which controls the color of the background panel it's parented to.
Output: 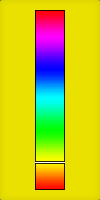
Example
Creates a DRGBPicker that controls the hue of a DColorCube, which outputs the color to the background panel, label, and your copy/paste buffer.
BGPanel
= vgui.
Create(
"DPanel")
BGPanel:
SetSize(
200,
200)
BGPanel:
Center()
local color_label
= Label(
"Color( 255, 255, 255 )", BGPanel)
color_label:
SetPos(
40,
160)
color_label:
SetSize(
150,
20)
color_label:
SetHighlight(
true)
color_label:
SetColor(
Color(
0,
0,
0))
local color_picker
= vgui.
Create(
"DRGBPicker", BGPanel)
color_picker:
SetPos(
5,
5)
color_picker:
SetSize(
30,
190)
local color_cube
= vgui.
Create(
"DColorCube", BGPanel)
color_cube:
SetPos(
40,
5)
color_cube:
SetSize(
155,
155)
function color_picker:
OnChange(col)
local h
= ColorToHSV(col)
local _, s, v
= ColorToHSV(
color_cube:
GetRGB())
col
= HSVToColor(h, s, v)
color_cube:
SetColor(col)
UpdateColors(col)
end
function color_cube:
OnUserChanged(col)
UpdateColors(col)
end
function UpdateColors(col)
BGPanel:
SetBackgroundColor(col)
color_label:
SetText(
"Color( "..col.r
..", "..col.g
..", "..col.b
.." )")
color_label:
SetColor(
Color((
255-col.
r), (
255-col.
g), (
255-col.b)))
SetClipboardText(
color_label:
GetText())
endOutput: 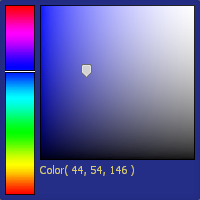