render.ClearStencilBufferRectangle
Example: Horizontal Wipe
This example demonstrates using this function to perform a "wipe" transition between two drawn elements.
Output: In the image below you can see the vertical split moving across the center of the screen revealing and hiding the two rectangles that are both being drawn in the same position.
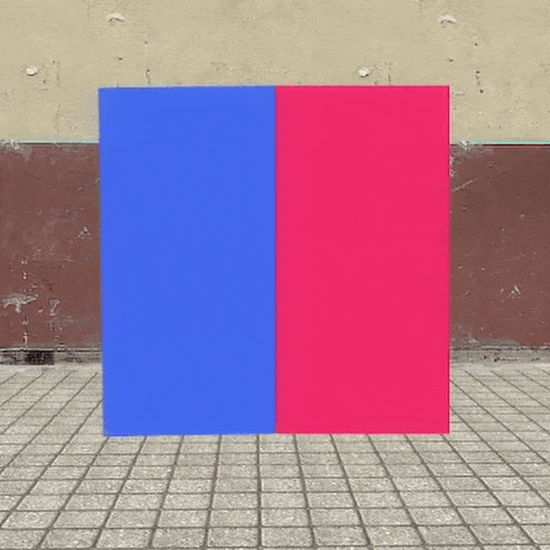
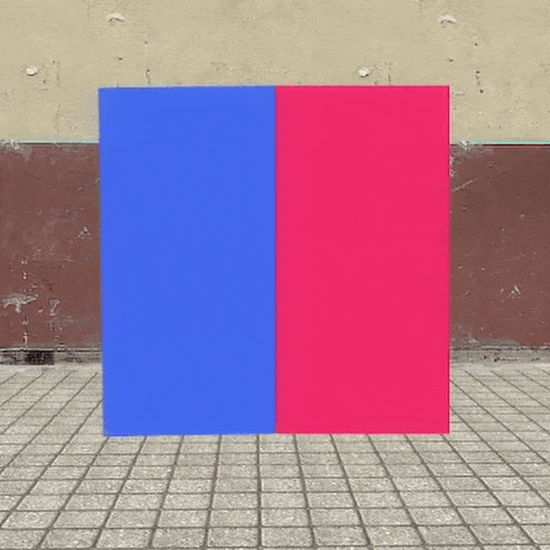