Panel:LocalToScreen
Example: Basic Usages
Here we create a DFrame derivative and use it to display the screen coordinates of the center of the panel. For and explanation of the design structure read VGUI Creating Custom Elements
Output: 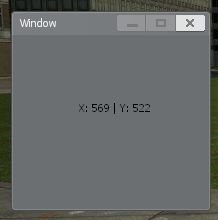
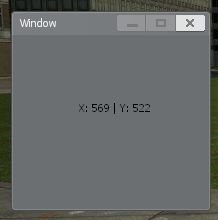
Takes X and Y coordinates relative to the panel and returns their corresponding positions relative to the screen.
See also Panel:ScreenToLocal.
Here we create a DFrame derivative and use it to display the screen coordinates of the center of the panel. For and explanation of the design structure read VGUI Creating Custom Elements