Entity:PhysicsInitMultiConvex
boolean Entity:PhysicsInitMultiConvex( table vertices, string surfaceprop = "default", Vector massCenterOverride = nil )
Description
An advanced version of Entity:PhysicsInitConvex which initializes a physics object from multiple convex meshes. This should be used for physics objects with a custom shape which cannot be represented by a single convex mesh.
If successful, the previous physics object will be removed.
Clientside physics objects are broken and do not move properly in some cases. Physics objects should only created on the server or you will experience incorrect physgun beam position, prediction issues, and other unexpected behavior.
A workaround is available on the Entity:PhysicsInitConvex page.
Issue Tracker: 5060
Arguments
1 table vertices
A table consisting of tables of Vectors. Each sub-table defines a set of points to be used in the computation of one convex mesh.
2 string surfaceprop = "default"
Physical material from surfaceproperties.txt or added with physenv.AddSurfaceData.
3 Vector massCenterOverride = nil
If set, overwrites the center of mass for the created physics object.
Returns
Example
Creates a physics mesh for the entity which consists of two boxes.
Output: 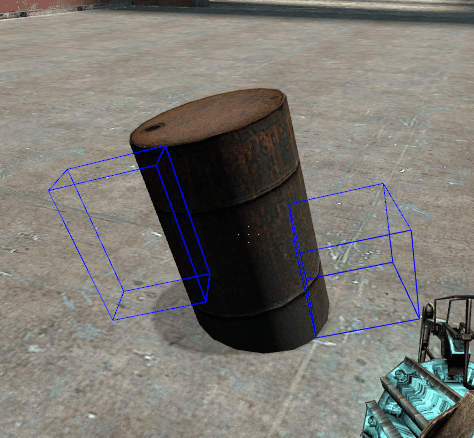
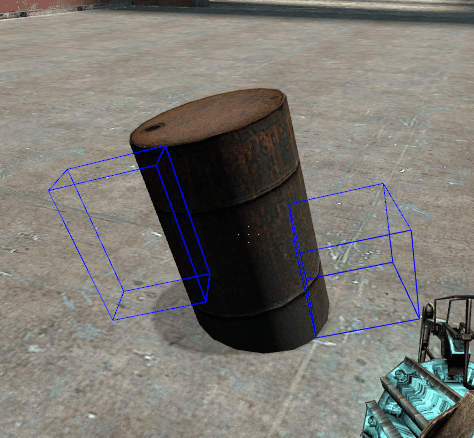