Entity:SetWeaponModel
Description
Sets the model and associated weapon to this viewmodel entity.
This is used internally when the player switches weapon.
View models are not drawn without a weapons associated to them.
This will silently fail if the entity is not a viewmodel.
Arguments
Example
Sets the model of the second viewmodel to the smg and associates it with the player's current weapon.
Example
Initializes the extra viewmodel in Deploy and hides it again on Holster, also plays the attack animation on left and right click.
Output: 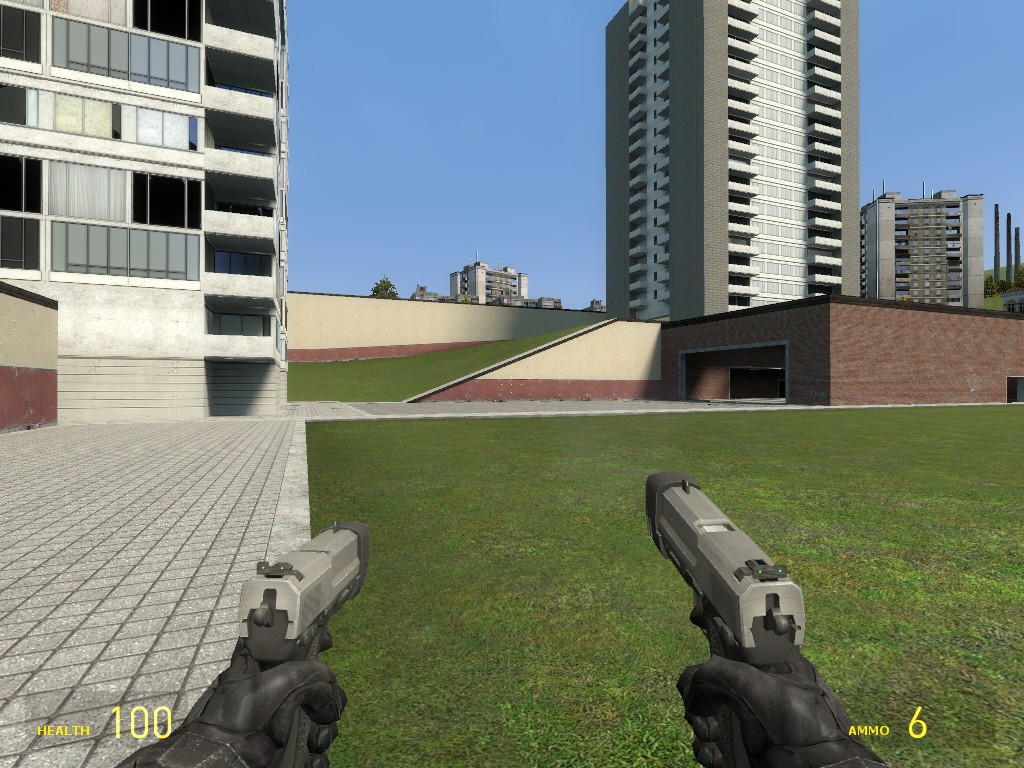
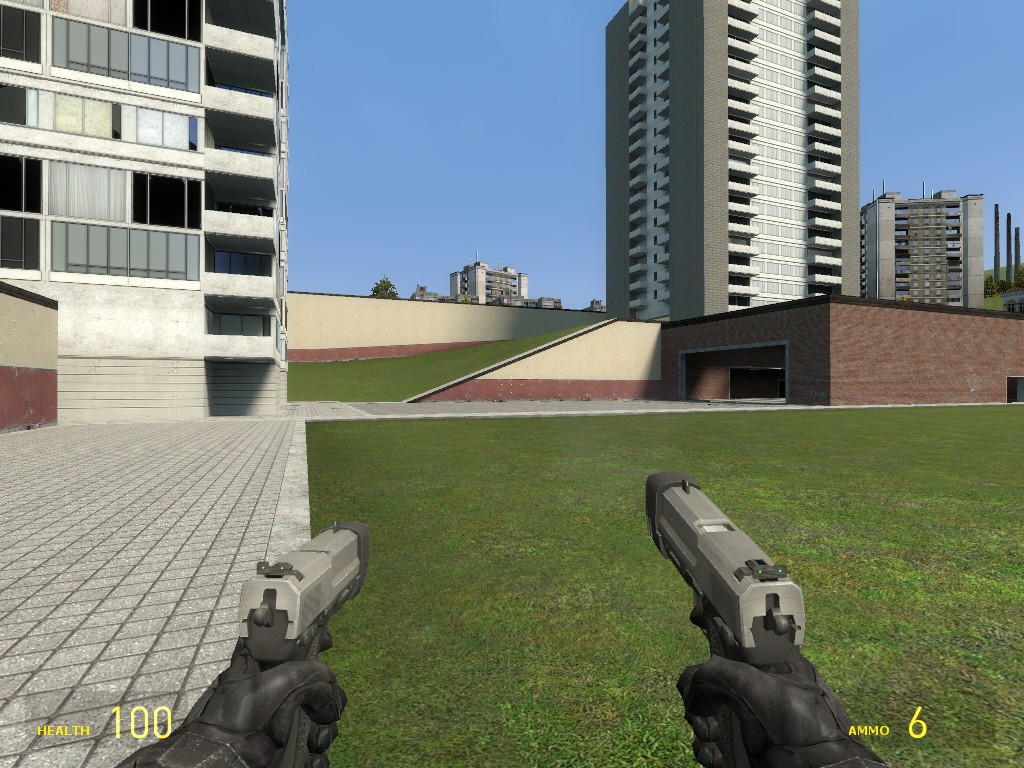