util.TraceHull
Description
Performs an AABB hull (axis-aligned bounding box, aka not rotated) trace with the given trace data.
This trace type cannot hit hitboxes.
See util.TraceLine for a simple line ("ray") trace.
This function may not always give desired results clientside due to certain physics mechanisms not existing on the client. Use it serverside for accurate results.
Arguments
Returns
Example
Visual representation of a Hull Trace.
Output: 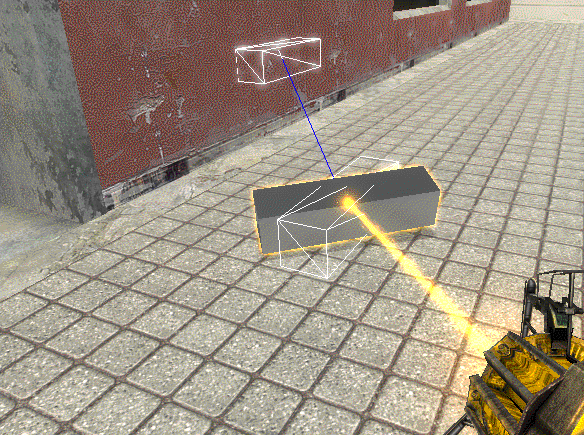
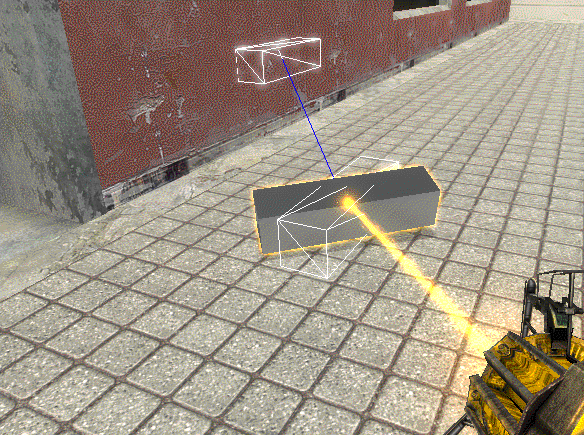
Example
Trace a player sized hull to detect if a player can spawn here without getting stuck inside anything.
Example
From a SWEP:PrimaryAttack()