surface.DrawPoly
Example
Draws a red triangle in the top left corner of the screen.
Output: 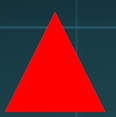
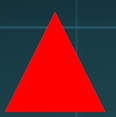
Example
Draws a yellow polygon in the top left corner of the screen.
Output: 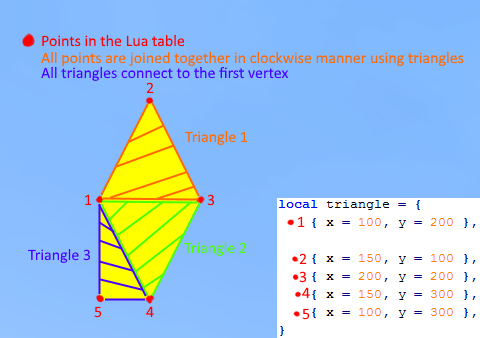
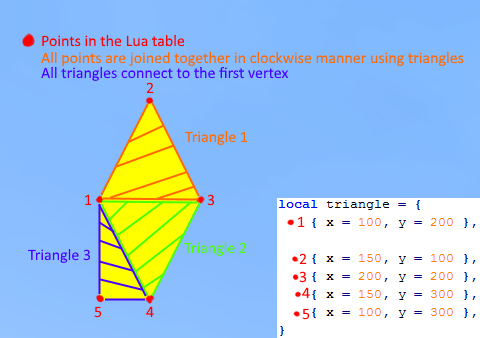
Example
A helper function to draw a circle using surface.DrawPoly.
Draws a textured polygon (secretly a triangle fan) with a maximum of 4096 vertices. Only works properly with convex polygons. You may try to render concave polygons, but there is no guarantee that things wont get messed up.
Unlike most surface library functions, non-integer coordinates are not rounded.
This means that it will only work in 2d Rendering Hooks.
The vertices must be in clockwise order.
Draws a red triangle in the top left corner of the screen.
Draws a yellow polygon in the top left corner of the screen.
A helper function to draw a circle using surface.DrawPoly.