DColorCube
Description
The DColorCube allows a user to select saturation and value but not hue. Uses HSV colors
Parent
Derives methods, etc not listed on this page from DSlider.
Implements
Implements or overrides the following hooks/methods. If you want to override these, you probably want to call the original function too.
Events
DColorCube:OnUserChanged( table color )
Function which is called when the color cube slider is moved (through user input). Meant to be overridden.
Methods
table DColorCube:GetBaseRGB()
An AccessorFunc that returns the base Color set by DColorCube:SetBaseRGB.
table DColorCube:GetDefaultColor()
An AccessorFunc that returns the color cube's default color. By default, it is set to white. (255 255 255 RGB)
We advise against using this. It may be changed or removed in a future update.
An AccessorFunc that returns the value set by DColorCube:SetHue.
DColorCube:ResetToDefaultValue()
Sets the color to whatever DColorCube:GetDefaultColor returns
DColorCube:SetBaseRGB( table color )
An AccessorFunc that sets the base color and the color used to draw the color cube panel itself.
Calling this when using a color that isn't 100% saturated and valued (HSVToColor with saturation and value set to 1) causes the color cube to look inaccurate compared to the color that's returned by methods like DColorCube:GetRGB and DColorCube:OnUserChanged. You should use DColorCube:SetColor instead
Sets the base color of the color cube and updates the slider position.
DColorCube:SetDefaultColor( table )
An AccessorFunc that sets the color cube's default color. This value will be used to reset to on middle mouse click of the color cube's draggable slider.
We advise against using this. It may be changed or removed in a future update.
An AccessorFunc that appears to do nothing and unused.
This is used internally - although you're able to use it you probably shouldn't.
An AccessorFunc that used internally to set the real "output" color of the panel.
This is used internally - although you're able to use it you probably shouldn't.
Updates the color cube RGB based on the given x and y position and returns its arguments. Similar to DColorCube:UpdateColor.
This is used internally - although you're able to use it you probably shouldn't.
Updates the color cube RGB based on the given x and y position. Similar to DColorCube:TranslateValues.
Example
Creates a DColorCube in a DFrame.
Example
Creates a color cube that's hue is controlled by a DRGBPicker, which outputs the color to the background panel, label, and your copy/paste buffer.
Output: 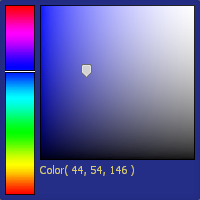
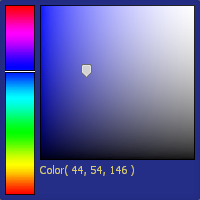