DFileBrowser
Description
A tree and list-based file browser.
It allows filtering by folder (directory) name and file extension, and can display models as SpawnIcons.
Parent
Derives methods, etc not listed on this page from DPanel.
Events
Called when a file is double-clicked.
Double-clicking a file or icon will trigger both this and DFileBrowser:OnSelect.
Called when a file is right-clicked.
When not in model viewer mode, DFileBrowser:OnSelect will also be called if the file is not already selected.
Methods
DFileBrowser:Clear()
Clears the file tree and list, and resets all values.
string DFileBrowser:GetBaseFolder()
Returns the root directory/folder of the file tree.
string DFileBrowser:GetCurrentFolder()
Returns the current directory/folder being displayed.
string DFileBrowser:GetFileTypes()
Returns the current file type filter on the file list.
Panel DFileBrowser:GetFolderNode()
Returns the DTree Node that the file tree stems from.
This is a child of the root node of the DTree.
Returns whether or not the model viewer mode is enabled. In this mode, files are displayed as SpawnIcons instead of a list.
Returns the access path of the file tree. This is GAME unless changed with DFileBrowser:SetPath.
See file. Read for how paths work.
DFileBrowser:SetBaseFolder( string baseDir )
Sets the root directory/folder of the file tree.
This needs to be set for the file tree to be displayed.
DFileBrowser:SetCurrentFolder( string currentDir )
Sets the directory/folder from which to display the file list.
DFileBrowser:SetFileTypes( string fileTypes = "." )
Sets the file type filter for the file list.
This accepts the same file extension wildcards as file. Find.
Enables or disables the model viewer mode. In this mode, files are displayed as SpawnIcons instead of a list.
This should only be used for . mdl files; the spawn icons will display error models for others. See DFileBrowser:SetFileTypes
Sets the access path for the file tree. This is set to GAME by default.
See file. Read for how paths work.
Sets the search filter for the file tree.
This accepts the same wildcards as file. Find.
This is used internally - although you're able to use it you probably shouldn't.
Called to set up the DTree and file viewer when a base path has been set.
Calls DFileBrowser:SetupTree and DFileBrowser:SetupFiles.
boolean DFileBrowser:SetupFiles()
This is used internally - although you're able to use it you probably shouldn't.
Called to set up the DListView or DIconBrowser by DFileBrowser:Setup.
The icon browser is used when in models mode. See DFileBrowser:SetModels.
This is used internally - although you're able to use it you probably shouldn't.
Called to set up the DTree by DFileBrowser:Setup.
DFileBrowser:ShowFolder( string currentDir )
This is used internally - although you're able to use it you probably shouldn't.
Builds the file or icon list for the current directory.
You should use DFileBrowser:SetCurrentFolder to change the directory.
Example
Creates a DFileBrowser and displays the data/persist folder. Any file clicked is printed to the console.
Output: 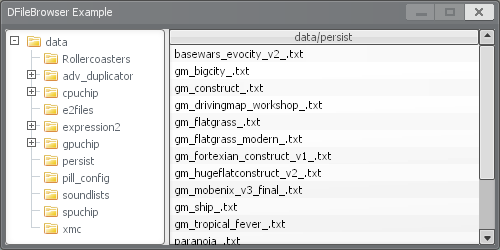
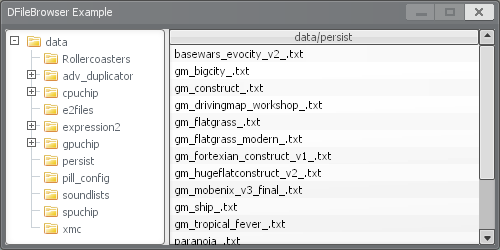
Example
Creates a DFileBrowser that can spawn models from props_
folders.
Uses the same DFrame as above
Output:
See Preview
.Example
Same as above, but enables model viewing. The following line is added to the above code.
Output: 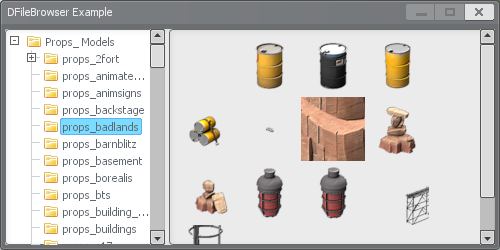
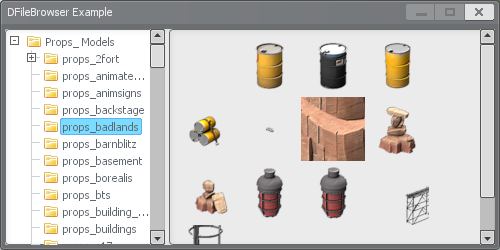
Example
Same as above, but enables model viewing. The following line is added to the above code.
Output: 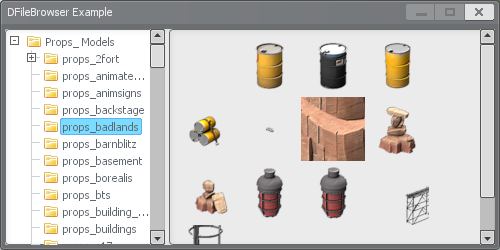
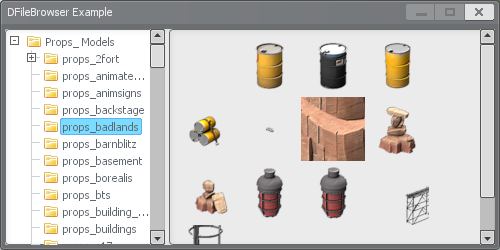
Example
Creates a DFileBrowser for browsing and selecting sounds.
Output: 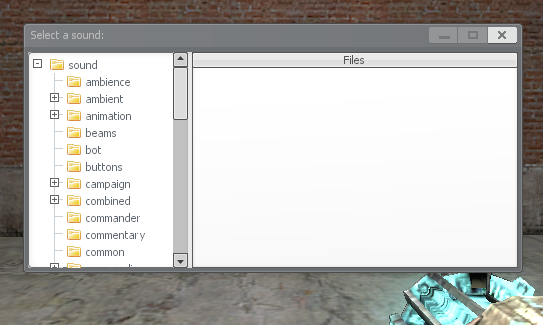
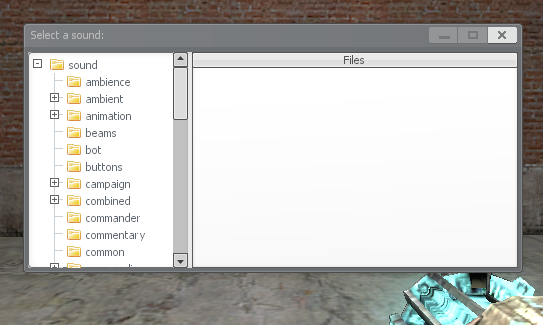