Panel:InsertColorChange
Example
Creates a RichText panel with color coding on certain segments of text.
Output: 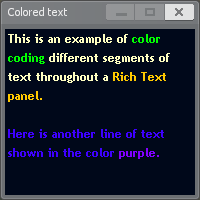
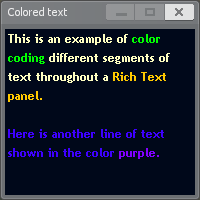
Example
Word by word coloring using string.Explode and random colors.
Output: 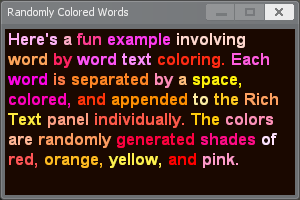
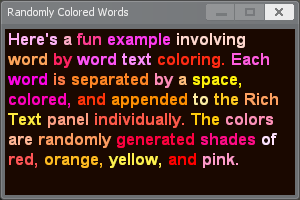
Inserts a color change in a RichText element, which affects the color of all text added with Panel:AppendText until another color change is applied.
Creates a RichText panel with color coding on certain segments of text.
Word by word coloring using string.Explode and random colors.