Description
Parent
Derives methods, etc not listed on this page from Button.
Implements
Implements or overrides the following hooks/methods. If you want to override these, you probably want to call the original function too.
Methods
Returns the checked state of the ImageCheckBox
Sets the checked state of the checkbox.
Checked state can be obtained by ImageCheckBox. State.
Sets the checked state of the checkbox.
Checked state can be obtained via ImageCheckBox:GetChecked
Sets the material that will be visible when the ImageCheckBox is checked.
Internally calls Material:SetMaterial.
Will error if no material was set.
Example
local DFrame
= vgui.
Create(
"DFrame" )
DFrame:
SetSize(
300,
305 )
DFrame:
Center()
DFrame:
SetTitle(
"Check list" )
DFrame:
MakePopup()
DFrame.Items
= {}
local Head
= DFrame:
Add(
"DPanel" )
Head:
Dock( TOP )
Head:
SetHeight(
20 )
function Head:
Paint( w, h )
local completed
= 0
for k, v
in ipairs( DFrame.Items )
do
if ( v.
ImageCheckBox:
GetChecked() )
then
completed
= completed
+ 1
end
end
draw.
SimpleText(
"You've completed " .. completed
.. " of " .. #DFrame.Items
.. " items",
"DermaDefaultBold", w
/ 2, h
/ 2,
Color(
255,
255,
255 ), TEXT_ALIGN_CENTER, TEXT_ALIGN_CENTER )
end
local function addItem( text )
local RulePanel
= DFrame:
Add(
"DPanel" )
RulePanel:
Dock( TOP )
RulePanel:
DockMargin(
0,
1,
0,
0 )
table.
insert( DFrame.Items, RulePanel )
local ImageCheckBox
= RulePanel:
Add(
"ImageCheckBox" )
ImageCheckBox:
SetMaterial(
"icon16/accept.png" )
ImageCheckBox:
SetWidth(
24 )
ImageCheckBox:
Dock( LEFT )
ImageCheckBox:
SetChecked(
false )
RulePanel.ImageCheckBox
= ImageCheckBox
local DLabel
= RulePanel:
Add(
"DLabel" )
DLabel:
SetText( text )
DLabel:
Dock( FILL )
DLabel:
DockMargin(
5,
0,
0,
0 )
DLabel:
SetTextColor(
Color(
0,
0,
0 ) )
end
addItem(
"Learn something" )
addItem(
"Do something" )
addItem(
"Make something" )
addItem(
"Create something" )
addItem(
"Play something" )
addItem(
"Test something" )
addItem(
"Write a really long item for testing purposes" )
addItem(
"Break something" )
addItem(
"Rebuild something" )
addItem(
"Release something" )
Output: 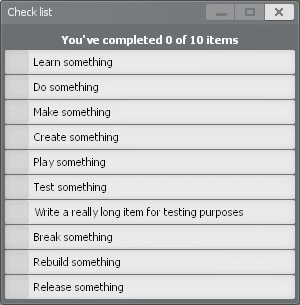