DModelPanel
Description
DModelPanel is a VGUI element that projects a 3D model onto a 2D plane. See also DAdjustableModelPanel
Parent
Derives methods, etc not listed on this page from DButton.
Events
DModelPanel:LayoutEntity( Entity entity )
By default, this function slowly rotates and animates the entity being rendered.
If you want to change this behavior, you should override it.
DModelPanel:PostDrawModel( Entity ent )
Called when the entity of the DModelPanel was drawn.
This is a rendering hook with 3d drawing context.
Called before the entity of the DModelPanel is drawn.
Methods
DModelPanel:DrawModel()
This is used internally - although you're able to use it you probably shouldn't.
Used by the DModelPanel's paint hook to draw the model and background.
table DModelPanel:GetAmbientLight()
Returns the ambient lighting used on the rendered entity.
boolean DModelPanel:GetAnimated()
Returns whether or not the panel entity should be animated when the default DModelPanel:LayoutEntity function is called.
number DModelPanel:GetAnimSpeed()
Returns the animation speed of the panel entity, see DModelPanel:SetAnimSpeed.
Angle DModelPanel:GetLookAng()
Returns the angles of the model viewing camera. Is nil until changed with DModelPanel:SetLookAng.
DModelPanel:RunAnimation()
This function is used in DModelPanel:LayoutEntity. It will progress the animation, set using Entity:SetSequence. By default, it is the walking animation.
DModelPanel:SetAmbientLight( table color )
Sets the ambient lighting used on the rendered entity.
DModelPanel:SetAnimated( boolean animated )
Sets whether or not to animate the entity when the default DModelPanel:LayoutEntity is called.
DModelPanel:SetAnimSpeed( number animSpeed )
Sets the speed used by DModelPanel:RunAnimation to advance frame on an entity sequence.
Entity:FrameAdvance doesn't seem to have any functioning arguments and therefore changing this will not have any affect on the panel entity's sequence speed without reimplementation. It only affects the value returned by DModelPanel:GetAnimSpeed
Sets the color of the rendered entity.
This does not work on Garry's Mod player models since they use a different color system. To modify a player model color, see Example 2 on the DModelPanel page
Sets the directional lighting used on the rendered entity.
Sets the entity to be rendered by the model panel.
If you set ent to a shared entity you must set ent to nil before removing this panel or else a "Trying to remove server entity on client!" error is thrown
DModelPanel:SetLookAng( Angle ang )
Sets the angles of the camera.
Makes the panel's camera face the given position. Basically sets the camera's angles (DModelPanel:SetLookAng) after doing some math.
Sets the model of the rendered entity.
This function may give a different model than expected. This is not a bug, however this problem may appear with some player models which are renamed several times in a wrong way. To solve that, you can use Entity:SetModel and Entity:SetModelName on the internal panel entity. More information : https://github. com/Facepunch/garrysmod-issues/issues/4534.
DModelPanel:StartScene( string path )
Runs a ClientsideScene on the panel's entity.
Example
Creates a DModelPanel and sets its model to your playermodel.
Example
Creates a DModelPanel and sets its model to the Alyx playermodel, then changes its player color to red. Also disables default rotation animation.
Output: 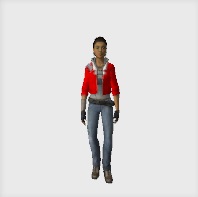
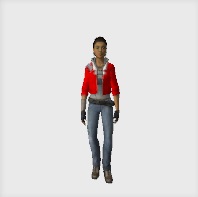