DListView
Description
A data view with rows and columns.
Parent
Derives methods, etc not listed on this page from DPanel.
Events
Called when a line in the DListView is double clicked.
Called when a row is right-clicked
Called internally by DListView:OnClickLine when a line is selected. This is the function you should override to define the behavior when a line is selected.
Methods
DListView:ClearSelection()
Clears the current selection in the DListView.
Gets the width of a column.
number DListView:DataLayout()
Creates the lines and gets the height of the contents, in a DListView.
DListView:DisableScrollbar()
Removes the scrollbar.
DListView:FixColumnsLayout()
This is used internally - although you're able to use it you probably shouldn't.
Internal helper function called from the PANEL:PerformLayout of DListView.
number DListView:GetDataHeight()
Returns the height of the data of the DListView.
See also DListView:SetDataHeight.
This is used internally - although you're able to use it you probably shouldn't.
See DListView:SetDirty.
number DListView:GetHeaderHeight()
Returns the height of the header of the DListView.
See also DListView:SetHeaderHeight.
boolean DListView:GetHideHeaders()
Returns whether the header line should be visible on not.
number DListView:GetInnerTall()
Returns the height of DListView:GetCanvas.
Intended to represent the height of all data lines.
boolean DListView:GetMultiSelect()
Returns whether multiple lines can be selected or not.
See DListView:SetMultiSelect.
table DListView:GetSelected()
Gets all of the lines that are currently selected.
Gets the currently selected DListView_Line index.
If DListView:SetMultiSelect is set to true, only the first line of all selected lines will be returned. Use DListView:GetSelected instead to get all of the selected lines.
boolean DListView:GetSortable()
Returns whether sorting of columns by clicking their headers is allowed or not.
See also DListView:SetSortable.
This is used internally - although you're able to use it you probably shouldn't.
Converts LineID to SortedID
This is used internally - although you're able to use it you probably shouldn't.
Use DListView:OnRowSelected instead!
Called whenever a line is clicked.
This is used internally - although you're able to use it you probably shouldn't.
Called from DListView_Column.
DListView:RemoveLine( number line )
Removes a line from the list view.
DListView:SelectFirstItem()
Selects the line at the first index of the DListView if one has been added.
DListView:SelectItem( Panel Line )
Selects a line in the listview.
DListView:SetDataHeight( number height )
Sets the height of all lines of the DListView except for the header line.
See also DListView:SetHeaderHeight.
This is used internally - although you're able to use it you probably shouldn't.
Used internally to signify if the DListView needs a rebuild.
DListView:SetHeaderHeight( number height )
Sets the height of the header line of the DListView.
See also DListView:SetDataHeight.
DListView:SetHideHeaders( boolean hide )
Sets whether the header line should be visible on not.
DListView:SetMultiSelect( boolean allowMultiSelect )
Sets whether multiple lines can be selected by the user by using the ctrl or ⇧ shift keys. When set to false, only one line can be selected.
DListView:SetSortable( boolean isSortable )
Enables/disables the sorting of columns by clicking.
This will only affect columns that are created after this function is called. Existing columns will be unaffected.
Sorts the items in the specified column.
Example
Creates a DListView and populates it with two columns and three items, only one of which can be selected at a time.
Selecting a row will print a console message containing the text of the row and its index.
Output:
Selected PesterChum ( 2mb ) at index 1
Selected Lumitorch ( 512kb ) at index 2
Selected Troj-on ( 661kb ) at index 3
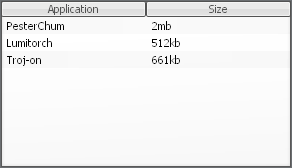