Render Library References
This page is part of the Render Reference, which is a set of pages dedicated to documenting the Render Library.
Other Render References pages include:
What are Beams?
The Beam functions make it easy to draw continuous lines in 3D space out of multiple connected Beam Segments.
Beam Segments are drawn out of textured quads that rotate to try to always face the camera (Billboards) in order to hide their flatness. They're used for things like the flame on thrusters, ropes, lightning effects, and more.
There are two approaches for drawing Beams:
- Straight-line Beams that only require a single Beam Segment can be easily drawn using:
- Continuous Beams that bend, curve, or otherwise require multiple Beam Segments can be drawn using:
Examples
Example: Multi-Segment
Drawing a star using a continuous Beam made out of multiple Beam Segments.
If you would like to run this example yourself, create a new folder in garrysmod/addons
called "RenderBeamExample" and place the sample code below into a .lua
file in garrysmod/addons/RenderBeamExample/lua/autorun/client/
Ensure that any other example code is removed or replaced to prevent unexpected behavior!
local beamWidth
= 10
local starRadius
= 100
local starCenter
= Vector(
0,
0,
0 )
local starTopLeft
= starCenter
+ Vector(
0,
math.
sin(
math.
rad(
-60 ) ),
math.
cos(
math.
rad(
-60 ) ) )
* starRadius
local starTopRight
= starCenter
+ Vector(
0,
math.
sin(
math.
rad(
60 ) ),
math.
cos(
math.
rad(
60 ) ) )
* starRadius
local starTopCenter
= starCenter
+ Vector(
0,
math.
sin(
math.
rad(
0 ) ),
math.
cos(
math.
rad(
0 ) ) )
* starRadius
local starBottomLeft
= starCenter
+ Vector(
0,
math.
sin(
math.
rad(
180 + 35 ) ),
math.
cos(
math.
rad(
180 + 35 ) ) )
* starRadius
local starBottomRight
= starCenter
+ Vector(
0,
math.
sin(
math.
rad(
180 - 35 ) ),
math.
cos(
math.
rad(
180 - 35 ) ) )
* starRadius
hook.
Add(
"PostDrawOpaqueRenderables",
"RenderBeamMultiSegmentExample",
function()
render.
SetColorMaterial()
render.
StartBeam(
6 )
render.
AddBeam( starBottomLeft, beamWidth,
0,
Color(
162,
224,
46 ) )
render.
AddBeam( starTopCenter, beamWidth,
0,
Color(
27,
189,
189 ) )
render.
AddBeam( starBottomRight, beamWidth,
0,
Color(
190,
38,
165 ) )
render.
AddBeam( starTopLeft, beamWidth,
0,
Color(
224,
132,
46 ) )
render.
AddBeam( starTopRight, beamWidth,
0,
Color(
207,
30,
30 ) )
render.
AddBeam( starBottomLeft, beamWidth,
0,
Color(
162,
224,
46 ) )
render.
EndBeam()
end )
Notice that where the Beam starts and ends (The bottom-left corner of the star) the Beam is the width specified in the calls to render.AddBeam. In the other corners of the star, the Beam appears thinner due to the end of the Beam being rotated to accomodate the next Beam Segment's direction. This is more easily seen in the wireframe view seen in the second screenshot below.
Example: Single-Segment
Drawing a star using several Beams made out of single Beam Segments.
If you would like to run this example yourself, create a new folder in garrysmod/addons
called "RenderBeamExample" and place the sample code below into a .lua
file in garrysmod/addons/RenderBeamExample/lua/autorun/client/
Ensure that any other example code is removed or replaced to prevent unexpected behavior!
local beamWidth
= 10
local starRadius
= 100
local starCenter
= Vector(
0,
0,
0 )
local starTopLeft
= starCenter
+ Vector(
0,
math.
sin(
math.
rad(
-60 ) ),
math.
cos(
math.
rad(
-60 ) ) )
* starRadius
local starTopRight
= starCenter
+ Vector(
0,
math.
sin(
math.
rad(
60 ) ),
math.
cos(
math.
rad(
60 ) ) )
* starRadius
local starTopCenter
= starCenter
+ Vector(
0,
math.
sin(
math.
rad(
0 ) ),
math.
cos(
math.
rad(
0 ) ) )
* starRadius
local starBottomLeft
= starCenter
+ Vector(
0,
math.
sin(
math.
rad(
180 + 35 ) ),
math.
cos(
math.
rad(
180 + 35 ) ) )
* starRadius
local starBottomRight
= starCenter
+ Vector(
0,
math.
sin(
math.
rad(
180 - 35 ) ),
math.
cos(
math.
rad(
180 - 35 ) ) )
* starRadius
hook.
Add(
"PostDrawOpaqueRenderables",
"RenderBeamSingleSegmentExample",
function()
render.
SetColorMaterial()
render.
DrawBeam( starBottomLeft, starTopCenter, beamWidth,
0,
1,
Color(
162,
224,
46 ) )
render.
DrawBeam( starTopCenter, starBottomRight, beamWidth,
0,
1,
Color(
27,
189,
189 ) )
render.
DrawBeam( starBottomRight, starTopLeft, beamWidth,
0,
1,
Color(
190,
38,
165 ) )
render.
DrawBeam( starTopLeft, starTopRight, beamWidth,
0,
1,
Color(
224,
132,
46 ) )
render.
DrawBeam( starTopRight, starBottomLeft, beamWidth,
0,
1,
Color(
207,
30,
30 ) )
end )
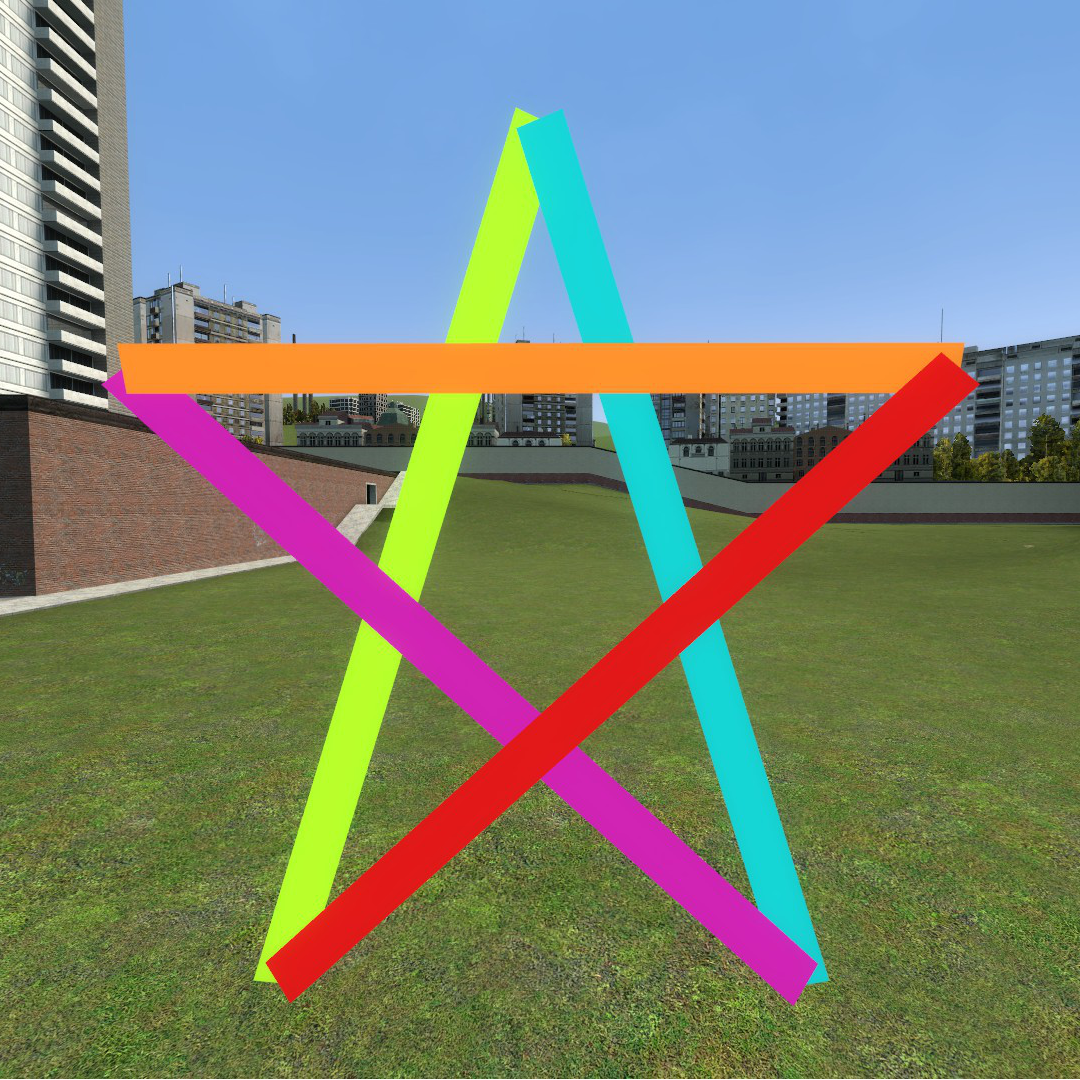
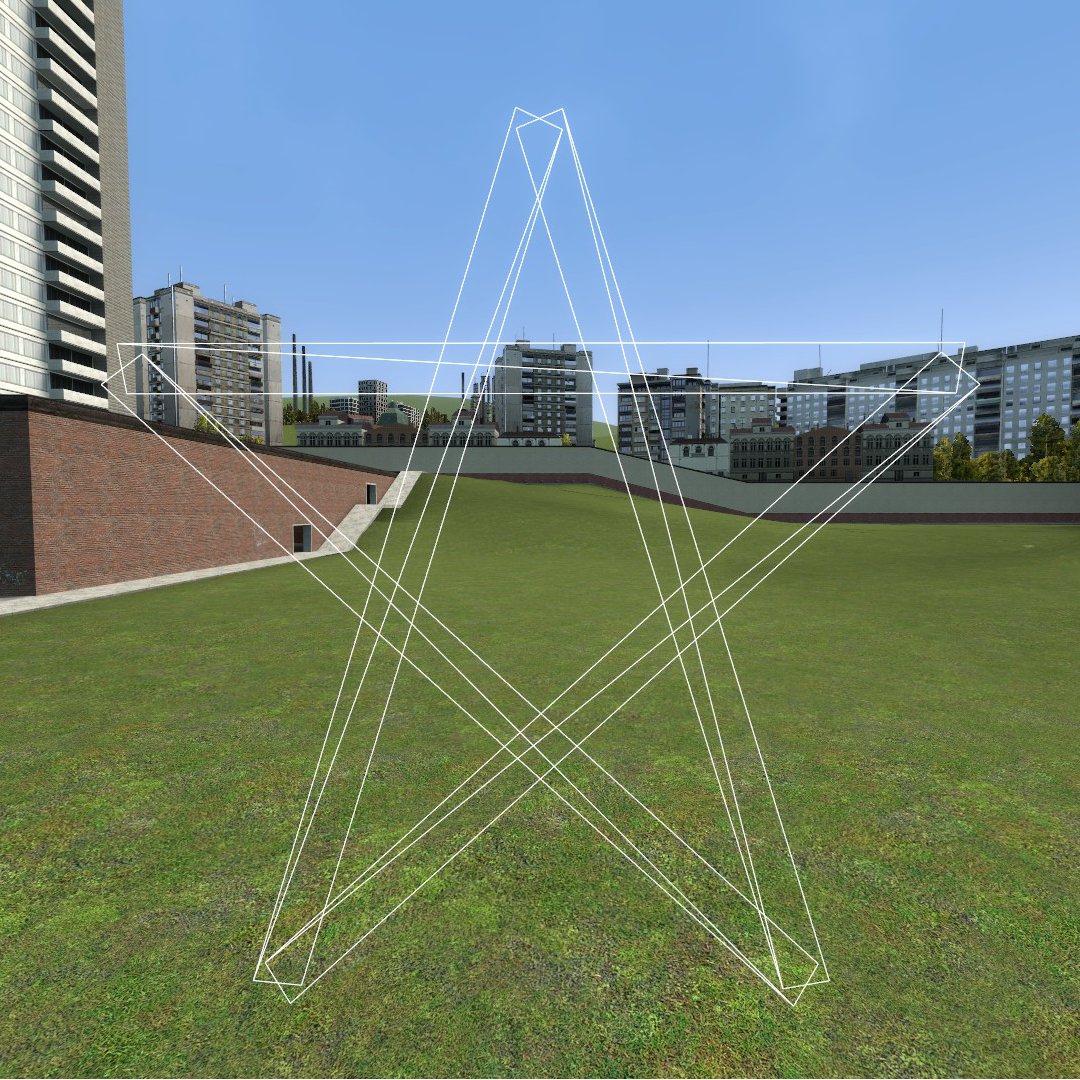